用swing写一个信息录 包含编号、姓名、性别、爱好、学历、电话,以表格形式
时间: 2023-07-04 10:15:31 浏览: 105
好的,下面是一个简单的信息录示例,使用Java Swing编写:
```
import javax.swing.*;
import java.awt.*;
import java.util.ArrayList;
public class InformationTable extends JFrame {
private JTable table;
private JScrollPane scrollPane;
private JButton addButton, deleteButton;
private JPanel buttonPanel;
private ArrayList<Person> personList;
public InformationTable() {
//设置窗口标题
setTitle("Information Table");
//创建表格
String[] columnNames = {"编号", "姓名", "性别", "爱好", "学历", "电话"};
Object[][] data = {};
table = new JTable(data, columnNames);
scrollPane = new JScrollPane(table);
//创建按钮
addButton = new JButton("Add Person");
deleteButton = new JButton("Delete Person");
//创建按钮面板
buttonPanel = new JPanel(new FlowLayout());
buttonPanel.add(addButton);
buttonPanel.add(deleteButton);
//创建人员列表
personList = new ArrayList<Person>();
//添加按钮监听器
addButton.addActionListener(e -> {
PersonDialog dialog = new PersonDialog(this, "Add Person");
dialog.setVisible(true);
});
deleteButton.addActionListener(e -> {
int selectedRow = table.getSelectedRow();
if (selectedRow != -1) {
int option = JOptionPane.showConfirmDialog(null, "Are you sure you want to delete this person?");
if (option == JOptionPane.YES_OPTION) {
personList.remove(selectedRow);
updateTable();
}
}
});
//添加表格和按钮面板到窗口
setLayout(new BorderLayout());
add(scrollPane, BorderLayout.CENTER);
add(buttonPanel, BorderLayout.SOUTH);
//设置窗口大小和可见性
setSize(600, 400);
setVisible(true);
}
//更新表格数据
public void updateTable() {
Object[][] data = new Object[personList.size()][6];
for (int i = 0; i < personList.size(); i++) {
Person person = personList.get(i);
data[i][0] = person.getId();
data[i][1] = person.getName();
data[i][2] = person.getGender();
data[i][3] = person.getHobby();
data[i][4] = person.getEducation();
data[i][5] = person.getPhone();
}
table.setModel(new javax.swing.table.DefaultTableModel(data, new String[]{"编号", "姓名", "性别", "爱好", "学历", "电话"}));
}
//添加人员
public void addPerson(Person person) {
personList.add(person);
updateTable();
}
public static void main(String[] args) {
new InformationTable();
}
}
class PersonDialog extends JDialog {
private JLabel idLabel, nameLabel, genderLabel, hobbyLabel, educationLabel, phoneLabel;
private JTextField idField, nameField, genderField, hobbyField, educationField, phoneField;
private JButton okButton, cancelButton;
private InformationTable parent;
public PersonDialog(InformationTable parent, String title) {
super(parent, title, true);
this.parent = parent;
//创建组件
idLabel = new JLabel("编号:");
nameLabel = new JLabel("姓名:");
genderLabel = new JLabel("性别:");
hobbyLabel = new JLabel("爱好:");
educationLabel = new JLabel("学历:");
phoneLabel = new JLabel("电话:");
idField = new JTextField(20);
nameField = new JTextField(20);
genderField = new JTextField(20);
hobbyField = new JTextField(20);
educationField = new JTextField(20);
phoneField = new JTextField(20);
okButton = new JButton("OK");
cancelButton = new JButton("Cancel");
//添加组件到面板
JPanel inputPanel = new JPanel(new GridLayout(6, 2));
inputPanel.add(idLabel);
inputPanel.add(idField);
inputPanel.add(nameLabel);
inputPanel.add(nameField);
inputPanel.add(genderLabel);
inputPanel.add(genderField);
inputPanel.add(hobbyLabel);
inputPanel.add(hobbyField);
inputPanel.add(educationLabel);
inputPanel.add(educationField);
inputPanel.add(phoneLabel);
inputPanel.add(phoneField);
JPanel buttonPanel = new JPanel(new FlowLayout());
buttonPanel.add(okButton);
buttonPanel.add(cancelButton);
//添加面板到窗口
setLayout(new BorderLayout());
add(inputPanel, BorderLayout.CENTER);
add(buttonPanel, BorderLayout.SOUTH);
//添加按钮监听器
okButton.addActionListener(e -> {
String id = idField.getText();
String name = nameField.getText();
String gender = genderField.getText();
String hobby = hobbyField.getText();
String education = educationField.getText();
String phone = phoneField.getText();
if (id.equals("") || name.equals("") || gender.equals("") || hobby.equals("") || education.equals("") || phone.equals("")) {
JOptionPane.showMessageDialog(null, "Please enter all fields.");
} else {
Person person = new Person(id, name, gender, hobby, education, phone);
parent.addPerson(person);
dispose();
}
});
cancelButton.addActionListener(e -> {
dispose();
});
//设置窗口大小和可见性
setSize(300, 300);
setLocationRelativeTo(parent);
}
}
class Person {
private String id, name, gender, hobby, education, phone;
public Person(String id, String name, String gender, String hobby, String education, String phone) {
this.id = id;
this.name = name;
this.gender = gender;
this.hobby = hobby;
this.education = education;
this.phone = phone;
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public String getGender() {
return gender;
}
public String getHobby() {
return hobby;
}
public String getEducation() {
return education;
}
public String getPhone() {
return phone;
}
}
```
这是一个具有添加和删除功能的信息录应用程序,以表格形式显示人员信息。用户可以添加新的人员并输入其编号、姓名、性别、爱好、学历和电话号码。应用程序使用Java的Swing库创建GUI。
阅读全文
相关推荐


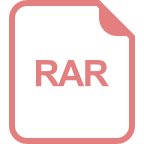
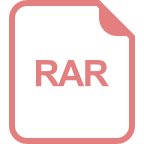
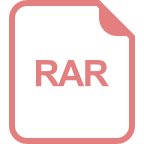
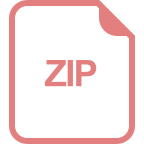
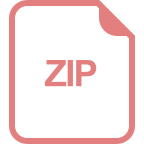
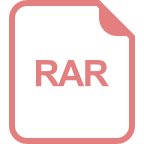
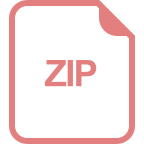
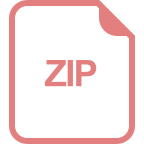
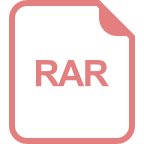
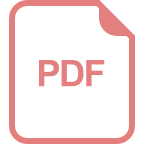
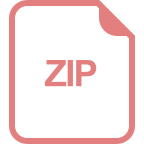
