c语言子串判断
时间: 2023-08-07 14:05:17 浏览: 253
在C语言中,可以使用库函数strstr来判断一个字符串是否为另一个字符串的子串。该函数原型为:
```c++
char *strstr(const char *str1, const char *str2);
```
其中,str1为原字符串,str2为要查找的子串。函数返回值为指向str2在str1中第一次出现的位置的指针,如果没有找到则返回NULL。
示例代码如下:
```c++
#include <stdio.h>
#include <string.h>
int main() {
char str1[20] = "hello world";
char str2[10] = "world";
if (strstr(str1, str2) != NULL) {
printf("%s is a substring of %s\n", str2, str1);
} else {
printf("%s is not a substring of %s\n", str2, str1);
}
return 0;
}
```
输出结果为:world is a substring of hello world
相关问题
c语言字符数组的子串判断
在C语言中,可以使用字符串处理函数`strstr()`来判断一个字符串是否包含另一个字符串,从而实现字符数组的子串判断。
`strstr()`函数的原型如下:
```c
char *strstr(const char *haystack, const char *needle);
```
其中,`haystack`表示要查找的字符串,`needle`表示要查找的子串。如果`haystack`中包含`needle`,则`strstr()`函数返回`haystack`中第一次出现`needle`的位置,否则返回`NULL`。
以下是一个例子,演示如何使用`strstr()`函数实现字符数组的子串判断:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str1[100] = "hello world";
char str2[100] = "world";
char *pos;
pos = strstr(str1, str2);
if (pos != NULL) {
printf("%s is a substring of %s\n", str2, str1);
} else {
printf("%s is not a substring of %s\n", str2, str1);
}
return 0;
}
```
输出结果为:
```
world is a substring of hello world
```
一个主串一个子串判断有无子串 C语言
可以使用C语言中的strstr函数来判断一个主串中是否包含一个子串。函数的原型为:
char *strstr(const char *haystack, const char *needle);
其中,haystack表示主串,needle表示要寻找的子串。如果找到了子串,函数返回指向该子串在主串中首次出现位置的指针;如果未找到,函数返回NULL。
阅读全文
相关推荐
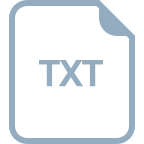
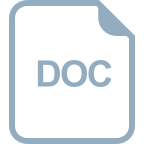






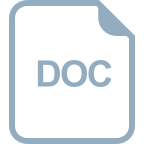





