spring security 默认的authentication-provider
时间: 2023-10-09 22:04:01 浏览: 145
Spring Security框架默认的authentication-provider是DaoAuthenticationProvider。DaoAuthenticationProvider可以从任何实现了UserDetailsService接口的地方获取用户信息,比如从内存中、数据库中或者LDAP中获取用户信息。在进行身份验证时,它会将用户提供的凭证与从UserDetailsService中获取的用户信息进行比较,以确定用户是否是合法用户。如果用户信息和提供的凭证匹配,则认证成功,否则认证失败。
相关问题
spring security 怎样 添加 自定义 authentication-provider 到 authentication-manager 中?
要将自定义的 `AuthenticationProvider` 添加到 `AuthenticationManager` 中,可以按照以下步骤进行操作:
1. 创建一个实现了 `AuthenticationProvider` 接口的类,并实现其中的 `authenticate` 方法。例如:
```java
public class MyAuthenticationProvider implements AuthenticationProvider {
@Override
public Authentication authenticate(Authentication authentication) throws AuthenticationException {
// 在这里进行身份认证逻辑的实现
}
@Override
public boolean supports(Class<?> authentication) {
// 返回该AuthenticationProvider支持的Authentication类型
return authentication.equals(UsernamePasswordAuthenticationToken.class);
}
}
```
2. 在 Spring 配置文件中配置 `AuthenticationManager` 和 `AuthenticationProvider`。例如:
```xml
<security:authentication-manager>
<security:authentication-provider ref="myAuthenticationProvider"/>
</security:authentication-manager>
<bean id="myAuthenticationProvider" class="com.example.MyAuthenticationProvider"/>
```
3. 现在你可以在你的代码中使用 `AuthenticationManager` 进行身份认证了。例如:
```java
@Autowired
private AuthenticationManager authenticationManager;
public void authenticateUser(String username, String password) {
Authentication authentication = new UsernamePasswordAuthenticationToken(username, password);
authenticationManager.authenticate(authentication);
}
```
在执行 `authenticationManager.authenticate(authentication)` 时,Spring Security 会自动调用 `MyAuthenticationProvider` 的 `authenticate` 方法进行身份认证。如果身份认证成功,则该方法会返回一个 `Authentication` 对象;如果身份认证失败,则会抛出一个 `AuthenticationException` 异常。
spring security 使用注解方式 添加 自定义 authentication-provider 到 authentication-manager 中?
要向Spring Security的`authentication-manager`中添加自定义的`authentication-provider`,可以通过注解方式实现。首先,需要创建一个实现了`AuthenticationProvider`接口的自定义类,例如:
```java
@Component
public class CustomAuthenticationProvider implements AuthenticationProvider {
@Override
public Authentication authenticate(Authentication authentication) throws AuthenticationException {
// 定义认证逻辑
}
@Override
public boolean supports(Class<?> authentication) {
return authentication.equals(UsernamePasswordAuthenticationToken.class);
}
}
```
然后,在任何一个配置类(如`WebSecurityConfigurerAdapter`)中,使用`@Autowired`注解将`CustomAuthenticationProvider`注入进来,并在`configure(AuthenticationManagerBuilder auth)`方法中添加它,如下所示:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private CustomAuthenticationProvider customAuthenticationProvider;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.authenticationProvider(customAuthenticationProvider);
}
// ...其他配置...
}
```
这样,`CustomAuthenticationProvider`就被添加到`authentication-manager`中了,当需要进行认证时,Spring Security会自动调用其`authenticate()`方法进行验证。
阅读全文
相关推荐










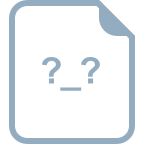




