用两个栈实现表达式求值c语言
时间: 2024-06-15 14:09:17 浏览: 143
在C语言中,可以使用两个栈来实现表达式求值。一个栈用于存储操作数,另一个栈用于存储操作符。
具体的实现步骤如下:
1. 创建两个栈,一个用于存储操作数,另一个用于存储操作符。
2. 从左到右遍历表达式的每个字符。
3. 如果当前字符是数字,则将其转换为操作数,并将其压入操作数栈。
4. 如果当前字符是操作符,则进行以下操作:
- 如果操作符栈为空,或者当前操作符的优先级大于栈顶操作符的优先级,则将当前操作符压入操作符栈。
- 否则,将栈顶操作符弹出,并从操作数栈中弹出两个操作数进行运算,将结果压入操作数栈。重复此过程直到满足条件后,将当前操作符压入操作符栈。
5. 如果当前字符是左括号"(",则将其压入操作符栈。
6. 如果当前字符是右括号")",则进行以下操作:
- 从操作符栈中弹出操作符,直到遇到左括号为止。同时从操作数栈中弹出两个操作数进行运算,将结果压入操作数栈。
- 弹出左括号"("。
7. 遍历完整个表达式后,如果操作符栈不为空,则从操作符栈中弹出操作符,并从操作数栈中弹出两个操作数进行运算,将结果压入操作数栈。
8. 最后,操作数栈中的唯一元素即为表达式的求值结果。
下面是一个用两个栈实现表达式求值的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
// 定义操作数栈和操作符栈
int operandStack[MAX_SIZE];
char operatorStack[MAX_SIZE];
int operandTop = -1;
int operatorTop = -1;
// 操作数栈的相关操作
void pushOperand(int operand) {
operandStack[++operandTop] = operand;
}
int popOperand() {
return operandStack[operandTop--];
}
// 操作符栈的相关操作
void pushOperator(char operator) {
operatorStack[++operatorTop] = operator;
}
char popOperator() {
return operatorStack[operatorTop--];
}
// 获取操作符的优先级
int getPriority(char operator) {
if (operator == '+' || operator == '-')
return 1;
else if (operator == '*' || operator == '/')
return 2;
else
return 0;
}
// 表达式求值函数
int evaluateExpression(char* expression) {
int i = 0;
while (expression[i] != '\0') {
if (expression[i] >= '0' && expression[i] <= '9') {
// 当前字符是数字,将其转换为操作数并压入操作数栈
int operand = 0;
while (expression[i] >= '0' && expression[i] <= '9') {
operand = operand * 10 + (expression[i] - '0');
i++;
}
pushOperand(operand);
} else if (expression[i] == '(') {
// 当前字符是左括号,将其压入操作符栈
pushOperator(expression[i]);
i++;
} else if (expression[i] == ')') {
// 当前字符是右括号,进行运算
while (operatorTop != -1 && operatorStack[operatorTop] != '(') {
char operator = popOperator();
int operand2 = popOperand();
int operand1 = popOperand();
int result;
switch (operator) {
case '+':
result = operand1 + operand2;
break;
case '-':
result = operand1 - operand2;
break;
case '*':
result = operand1 * operand2;
break;
case '/':
result = operand1 / operand2;
break;
}
pushOperand(result);
}
// 弹出左括号
popOperator();
i++;
} else {
// 当前字符是操作符,进行运算
while (operatorTop != -1 && getPriority(expression[i]) <= getPriority(operatorStack[operatorTop])) {
char operator = popOperator();
int operand2 = popOperand();
int operand1 = popOperand();
int result;
switch (operator) {
case '+':
result = operand1 + operand2;
break;
case '-':
result = operand1 - operand2;
break;
case '*':
result = operand1 * operand2;
break;
case '/':
result = operand1 / operand2;
break;
}
pushOperand(result);
}
// 将当前操作符压入操作符栈
pushOperator(expression[i]);
i++;
}
}
// 进行最后的运算
while (operatorTop != -1) {
char operator = popOperator();
int operand2 = popOperand();
int operand1 = popOperand();
int result;
switch (operator) {
case '+':
result = operand1 + operand2;
break;
case '-':
result = operand1 - operand2;
break;
case '*':
result = operand1 * operand2;
break;
case '/':
result = operand1 / operand2;
break;
}
pushOperand(result);
}
// 返回最终结果
return operandStack[operandTop];
}
int main() {
char expression[MAX_SIZE];
printf("请输入表达式:");
scanf("%s", expression);
int result = evaluateExpression(expression);
printf("表达式的求值结果为:%d\n", result);
return 0;
}
```
阅读全文
相关推荐





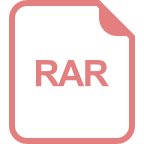









