如何在子线程中获取ThreadLocal
时间: 2023-07-26 07:16:12 浏览: 119
在Java中,每个线程都有自己的ThreadLocal变量副本,可以在不同的线程中访问ThreadLocal中的数据,而不会出现线程安全问题。如果在子线程中需要获取ThreadLocal中的数据,可以通过以下两种方式来实现:
1. 将ThreadLocal对象传递给子线程:在创建子线程时,将主线程中的ThreadLocal对象作为参数传递给子线程,在子线程中就可以访问到主线程中的ThreadLocal对象,并获取其中的数据。
示例代码如下:
```java
public class MyRunnable implements Runnable {
private ThreadLocal<String> threadLocal;
public MyRunnable(ThreadLocal<String> threadLocal) {
this.threadLocal = threadLocal;
}
public void run() {
String data = threadLocal.get(); // 在子线程中获取ThreadLocal中的数据
// do something with data
}
}
// 在主线程中创建ThreadLocal对象
ThreadLocal<String> threadLocal = new ThreadLocal<>();
threadLocal.set("data");
// 在主线程中创建子线程,并将ThreadLocal对象传递给子线程
Thread thread = new Thread(new MyRunnable(threadLocal));
thread.start();
```
2. 使用InheritableThreadLocal对象:InheritableThreadLocal对象是ThreadLocal的一个子类,可以实现将ThreadLocal变量值从父线程传递到子线程中。
示例代码如下:
```java
public class MyRunnable implements Runnable {
private InheritableThreadLocal<String> threadLocal;
public MyRunnable(InheritableThreadLocal<String> threadLocal) {
this.threadLocal = threadLocal;
}
public void run() {
String data = threadLocal.get(); // 在子线程中获取ThreadLocal中的数据
// do something with data
}
}
// 在主线程中创建InheritableThreadLocal对象
InheritableThreadLocal<String> threadLocal = new InheritableThreadLocal<>();
threadLocal.set("data");
// 在主线程中创建子线程,子线程可以继承ThreadLocal对象中的数据
Thread thread = new Thread(new MyRunnable(threadLocal));
thread.start();
```
需要注意的是,ThreadLocal中保存的数据只在当前线程中有效,如果需要在多个线程中共享数据,需要使用其他的方式来实现,例如使用静态变量、全局变量、单例模式、数据库、缓存、消息队列等方式。
阅读全文
相关推荐
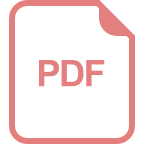
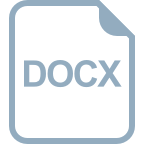



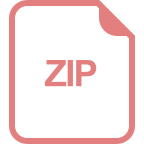
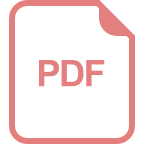
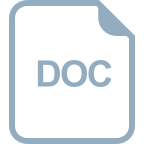
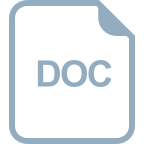
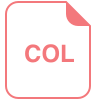






