pyside6结合yolov5
时间: 2023-11-06 18:06:43 浏览: 76
pyside6是一个Python的GUI框架,而yolov5是一个目标检测算法。它们之间并没有直接的联系,但是可以通过编写代码将它们结合起来使用。
具体来说,可以使用pyside6编写一个GUI界面,让用户选择一张图片,然后将这张图片输入到yolov5模型中进行目标检测,最后将检测结果显示在GUI界面上。
以下是一个简单的示例代码:
```python
import sys
from PySide6.QtWidgets import QApplication, QMainWindow, QLabel, QPushButton, QFileDialog
from PySide6.QtGui import QPixmap
import torch
import cv2
from models.experimental import attempt_load
from utils.general import non_max_suppression, scale_coords
from utils.plots import plot_one_box
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建GUI界面上的控件
self.label = QLabel(self)
self.label.setGeometry(50, 50, 400, 400)
self.button = QPushButton('选择图片', self)
self.button.setGeometry(200, 500, 100, 50)
self.button.clicked.connect(self.select_image)
# 加载yolov5模型
self.model = attempt_load('yolov5s.pt', map_location=torch.device('cpu'))
def select_image(self):
# 打开文件选择对话框,让用户选择一张图片
file_path, _ = QFileDialog.getOpenFileName(self, '选择图片', '', 'Images (*.png *.xpm *.jpg)')
# 加载图片并进行目标检测
img = cv2.imread(file_path)
results = self.detect(img)
# 在图片上绘制检测结果
for x1, y1, x2, y2, conf, cls in results:
plot_one_box((x1, y1, x2, y2), img, label=cls, color=(0, 255, 0))
# 将图片显示在GUI界面上
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
h, w, c = img.shape
qimg = QPixmap.fromImage(QImage(img.data, w, h, c * w, QImage.Format_RGB888))
self.label.setPixmap(qimg)
def detect(self, img):
# 对图片进行预处理
img = cv2.resize(img, (640, 640))
img = img[:, :, ::-1].transpose(2, 0, 1)
img = torch.from_numpy(img).float() / 255.0
img = img.unsqueeze(0)
# 使用yolov5模型进行目标检测
with torch.no_grad():
outputs = self.model(img)
results = non_max_suppression(outputs['pred'], conf_thres=0.5)
# 将检测结果转换为opencv格式
results = results[0].cpu().numpy()
results[:, :4] = scale_coords(img.shape[2:], results[:, :4], img.shape[2:]).round()
return results
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
在这个示例代码中,我们创建了一个MainWindow类,继承自QMainWindow类,用于显示GUI界面。在GUI界面上,我们创建了一个QLabel控件用于显示图片,一个QPushButton控件用于选择图片。当用户点击选择图片按钮时,会弹出文件选择对话框,让用户选择一张图片。然后我们调用detect方法对这张图片进行目标检测,并将检测结果绘制在图片上,最后将图片显示在GUI界面上。
相关推荐
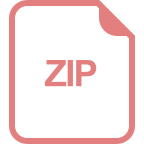
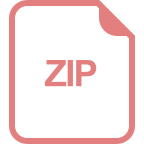














