yolov8-pyside6-gui-main
时间: 2023-11-28 18:02:48 浏览: 77
yolov8-pyside6-gui-main是一个基于YOLOv8目标检测模型和PySide6 GUI库的主要代码文件或项目名称。YOLOv8是一种先进的目标检测模型,能够快速准确地检测图像或视频中的多个对象。而PySide6是一个用于创建交互式应用程序的Python模块,它提供了丰富的图形用户界面功能。
yolov8-pyside6-gui-main可能是一个结合了YOLOv8模型和PySide6库的项目,旨在开发一个具有图形用户界面的目标检测应用程序。它可能包括了使用YOLOv8模型进行对象检测的算法实现,同时也使用PySide6来创建用户友好的GUI界面,让用户能够通过简单的操作来使用目标检测功能。
这个项目可能包括了图像或视频的输入功能,用户可以通过GUI界面选择要进行目标检测的文件,同时也提供了可视化的结果展示,让用户能够直观地了解检测到的对象信息。此外,该项目可能还包括了其他功能,比如设置检测的阈值、选择不同的检测模型、保存检测结果等。
总之,yolov8-pyside6-gui-main可能是一个结合了目标检测算法和图形用户界面技术的项目,旨在为用户提供一个便捷、直观的目标检测应用程序。
相关问题
yolov5-pyside调用海康摄像检测
很抱歉,根据提供的引用内容,没有直接回答您关于yolov5-pyside调用海康摄像检测的问题。但是,我们可以给您提供一些相关信息,帮助您更好地解决问题。
引用[1]中提到,Qt版本存在不兼容的情况,需要自己亲自安装各个版本编译运行并测试。因此,如果您使用yolov5-pyside调用海康摄像头时遇到了问题,可能需要考虑Qt版本的兼容性问题。
引用[2]中也提到了,在Qt官方新增的BUG方面,可以通过查询Qt官方BUG网站来解决问题。因此,如果您遇到问题,可以尝试在Qt官方BUG网站上查询是否有相关解决方案。
此外,我们建议您查阅相关文献或者咨询专业人士,以便更好地解决问题。
--相关问题--:
python-pyside6
好的,你的问题是 "python-pyside6",我理解为你想了解关于 PySide6 的 Python 库的信息。PySide6 是一个用于创建跨平台桌面应用程序的 Python 模块,它是 Qt 应用程序框架的 Python 绑定。PySide6 提供了一组 Python 模块,可以让开发者使用 Python 语言来编写 Qt 应用程序。
PySide6 支持 Qt 6,并且与 Python 3.7、3.8、3.9 兼容。它提供了许多 Qt 模块的 Python 封装,包括 QtCore、QtGui、QtWidgets、QtNetwork、QtXml、QtSvg 等等。使用 PySide6,开发者可以使用 Python 语言来创建跨平台的桌面应用程序,而无需学习 C++。
相关推荐
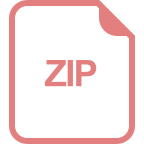
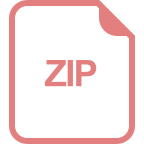
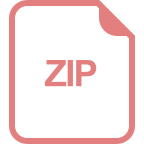












