c语言编写单月的日历
时间: 2024-11-08 15:10:24 浏览: 21
在C语言中编写一个简单的单月日历程序,通常会涉及到日期计算、月份天数以及输出格式化。以下是一个基本的示例,展示如何创建一个函数来生成指定月份的日历:
```c
#include <stdio.h>
#include <stdlib.h>
// 函数声明
void printCalendar(int year, int month);
// 定义闰年判断函数
int is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
// 计算指定月份的天数
int days_in_month(int month, int year) {
if(month == 2) {
return is_leap_year(year) ? 29 : 28;
}
switch(month) {
case 4:
case 6:
case 9:
case 11:
return 30;
default:
return 31;
}
}
// 打印日历函数
void printCalendar(int year, int month) {
const char* months[] = {"January", "February", "March", "April", "May", "June",
"July", "August", "September", "October", "November", "December"};
int i, j;
printf("%s %d\n", months[month - 1], year);
printf("------------------------\n");
for(i = 1; i <= days_in_month(month, year); ++i) {
j = (i < 10) ? (' ' * (2 - i)) + i : i;
printf("%2d", j);
if((j == 7 || i == days_in_month(month, year)) && i != 7) {
printf("\n");
} else {
printf(" ");
}
}
printf("\n");
}
int main() {
int year, month;
printf("Enter the year and month (1-12): ");
scanf("%d %d", &year, &month);
if(month >= 1 && month <= 12) {
printCalendar(year, month);
} else {
printf("Invalid month!\n");
}
return 0;
}
```
在这个例子中,用户会被提示输入年份和月份,然后`printCalendar`函数会打印出该月的日历。注意,这个代码简化处理了边界情况,例如没有检查输入是否为闰年。
阅读全文
相关推荐
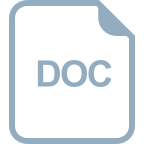
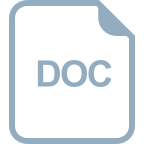
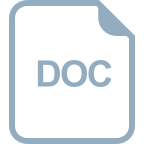
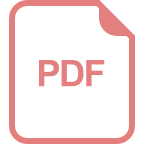
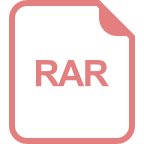
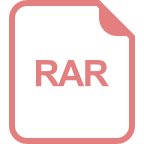
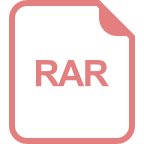
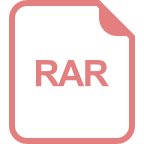
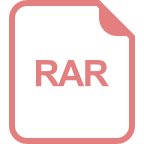
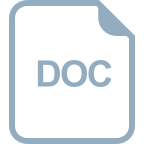


