python任意输入一串字符,输出其中不同字符以及各自的个数。例如,输入“abcdefgabc”,输出为a->2,b->2,c->2,d->1,e->1,f->1,g->1。
时间: 2023-05-31 20:19:35 浏览: 123
### 回答1:
可以使用 Python 中的字典来解决这个问题。遍历输入的字符串,对于每个字符,若字典中已有记录则该字符对应的值加 1,否则将该字符作为键加入字典,并将对应的值初始化为 1。最后遍历字典输出键值对即可。
代码如下:
```
s = input()
d = {}
for c in s:
if c in d:
d[c] += 1
else:
d[c] = 1
for c, count in d.items():
print(c + '->' + str(count))
```
例如输入字符串为 "abcdefgabc",输出结果为:
```
a->2
b->2
c->2
d->1
e->1
f->1
g->1
```
### 回答2:
Python有很多种方法可以实现统计字符串中不同字符的出现次数的功能。以下是一种简单的方法:
1. 首先输入一串字符串,可以使用input()函数来实现:
```python
string = input("请输入一串字符:")
```
2. 接下来,定义一个字典来存储字符和它们的出现次数,然后遍历每一个字符,将它们添加到字典中:
```python
char_dict = {}
for char in string:
if char in char_dict:
char_dict[char] += 1
else:
char_dict[char] = 1
```
3. 最后,遍历字典,将每个字符以及它们的出现次数打印出来:
```python
for char, count in char_dict.items():
print(char + "->" + str(count))
```
完整代码如下:
```python
string = input("请输入一串字符:")
char_dict = {}
for char in string:
if char in char_dict:
char_dict[char] += 1
else:
char_dict[char] = 1
for char, count in char_dict.items():
print(char + "->" + str(count))
```
以上代码可以输出每个字符出现的次数,如果想要按照字符的首次出现顺序来输出,可以将char_dict定义为有序字典,例如:
```python
from collections import OrderedDict
string = input("请输入一串字符:")
char_dict = OrderedDict()
for char in string:
if char in char_dict:
char_dict[char] += 1
else:
char_dict[char] = 1
for char, count in char_dict.items():
print(char + "->" + str(count))
```
这样输出的字符就会按照输入顺序来排列。
### 回答3:
要实现输入一串字符,输出其中不同字符以及各自的个数,可以用Python语言编写一个简单的程序。逻辑很简单:遍历每个字符,将每个字符统计出现次数,并输出。
具体实现步骤如下:
1. 通过 input 函数获取用户输入的一串字符;
2. 初始化一个字典,用于记录每个字符出现的次数;
3. 遍历之前输入的字符串,统计每个字符出现的次数,并存入字典;
4. 遍历字典,输出每个不同字符以及对应的出现次数。
下面是一个可以实现上述逻辑的 Python 代码:
```python
input_str = input("请输入一串字符:")
count_dict = {}
for char in input_str:
if char not in count_dict:
count_dict[char] = 1
else:
count_dict[char] += 1
for char, count in count_dict.items():
print(f"{char}->{count}")
```
代码运行效果如下:
```plain
请输入一串字符:abcdefgabc
a->2
b->2
c->2
d->1
e->1
f->1
g->1
```
以上就是简单实现 Python 输入一串字符,输出其中不同字符以及各自的个数的方法了。实际应用中,这种统计字符出现次数的需求也是比较常见的。
相关推荐
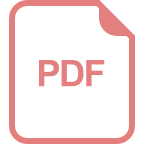
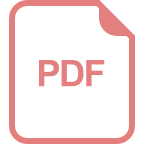
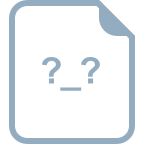














