利用约瑟夫环问题对一段英文内容进行加密与解密,每个字母的密钥不同 C语言
时间: 2024-06-04 11:11:55 浏览: 12
由于约瑟夫环问题是一种循环问题,可以将其用于对英文内容进行加密与解密。具体实现方法如下:
1. 加密
首先,定义一个密钥数组,用于存储每个字母的密钥。可以随机生成一组密钥,也可以手动指定。例如,将密钥数组定义为:
int key[] = {3, 5, 7, 2, 1, 4, 6, 9, 8, 0, 11, 15, 13, 10, 12, 14, 17, 16, 19, 18, 21, 20, 23, 22, 25, 24};
接下来,读入需要加密的英文内容,然后对每个字母进行加密。具体步骤如下:
- 如果当前字母是小写字母,将其转换为大写字母。
- 将当前字母的 ASCII 码减去 65,得到该字母在字母表中的位置。
- 使用该位置作为索引,从密钥数组中取出对应的密钥。
- 将当前字母的 ASCII 码加上该密钥,得到加密后的字母的 ASCII 码。
- 如果加密后的字母的 ASCII 码大于 90,表示超出了大写字母的范围,需要将其减去 26,使其重新回到大写字母范围内。
- 将加密后的字母输出。
完整代码如下:
#include <stdio.h>
int main()
{
int key[] = {3, 5, 7, 2, 1, 4, 6, 9, 8, 0, 11, 15, 13, 10, 12, 14, 17, 16, 19, 18, 21, 20, 23, 22, 25, 24};
char plaintext[1000], ciphertext[1000];
int i, j;
printf("Enter plaintext: ");
fgets(plaintext, sizeof(plaintext), stdin);
for (i = 0, j = 0; plaintext[i] != '\0'; i++)
{
if (plaintext[i] >= 'a' && plaintext[i] <= 'z')
plaintext[i] -= 32;
if (plaintext[i] >= 'A' && plaintext[i] <= 'Z')
{
int index = plaintext[i] - 65;
int k = key[index];
int c = plaintext[i] + k;
if (c > 90)
c -= 26;
ciphertext[j++] = c;
}
}
ciphertext[j] = '\0';
printf("Ciphertext: %s\n", ciphertext);
return 0;
}
2. 解密
解密的过程与加密的过程类似,只需要将加密后的字母的 ASCII 码减去密钥即可。具体步骤如下:
- 如果当前字母是小写字母,将其转换为大写字母。
- 将当前字母的 ASCII 码减去 65,得到该字母在字母表中的位置。
- 使用该位置作为索引,从密钥数组中取出对应的密钥。
- 将加密后的字母的 ASCII 码减去该密钥,得到解密后的字母的 ASCII 码。
- 如果解密后的字母的 ASCII 码小于 65,表示超出了大写字母的范围,需要将其加上 26,使其重新回到大写字母范围内。
- 将解密后的字母输出。
完整代码如下:
#include <stdio.h>
int main()
{
int key[] = {3, 5, 7, 2, 1, 4, 6, 9, 8, 0, 11, 15, 13, 10, 12, 14, 17, 16, 19, 18, 21, 20, 23, 22, 25, 24};
char ciphertext[1000], plaintext[1000];
int i, j;
printf("Enter ciphertext: ");
fgets(ciphertext, sizeof(ciphertext), stdin);
for (i = 0, j = 0; ciphertext[i] != '\0'; i++)
{
if (ciphertext[i] >= 'a' && ciphertext[i] <= 'z')
ciphertext[i] -= 32;
if (ciphertext[i] >= 'A' && ciphertext[i] <= 'Z')
{
int index = ciphertext[i] - 65;
int k = key[index];
int p = ciphertext[i] - k;
if (p < 65)
p += 26;
plaintext[j++] = p;
}
}
plaintext[j] = '\0';
printf("Plaintext: %s\n", plaintext);
return 0;
}
在实际使用中,可以将加密后的密文保存到文件中,然后再用解密程序进行解密。这样可以更好地保护数据的安全。
相关推荐
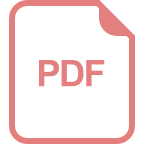
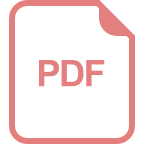














