利用列表解决约瑟夫环问题c语言
时间: 2023-12-02 11:24:47 浏览: 87
好的,下面是利用链表解决约瑟夫环问题的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct Node {
int data;
struct Node* next;
};
// 创建链表
struct Node* createList(int n) {
struct Node* head = NULL;
struct Node* tail = NULL;
for (int i = 1; i <= n; i++) {
struct Node* node = (struct Node*)malloc(sizeof(struct Node));
node->data = i;
node->next = NULL;
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
tail->next = head; // 将尾节点指向头节点,形成环形链表
return head;
}
// 模拟约瑟夫环过程
void josephus(int m, struct Node** head) {
struct Node* curr = *head;
struct Node* prev = NULL;
while (curr->next != curr) {
// 找到要删除的节点
for (int i = 1; i < m; i++) {
prev = curr;
curr = curr->next;
}
// 删除节点
prev->next = curr->next;
printf("%d ", curr->data);
free(curr);
curr = prev->next;
}
*head = curr; // 更新头节点
}
int main() {
int n, m;
printf("请输入总人数n和报数m:");
scanf("%d %d", &n, &m);
struct Node* head = createList(n);
printf("出列的顺序为:");
josephus(m, &head);
printf("%d\n", head->data);
return 0;
}
```
该程序中,我们首先定义了一个链表节点结构体`Node`,然后通过`createList`函数创建了一个包含`n`个节点的环形链表,用来模拟约瑟夫环。接着,我们定义了`josephus`函数,该函数用来模拟约瑟夫环的出列过程。最后,在`main`函数中,我们让用户输入总人数`n`和报数`m`,然后调用`josephus`函数模拟约瑟夫环的出列过程,最终输出出列的顺序。
阅读全文
相关推荐
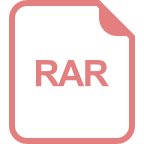
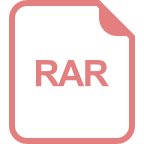
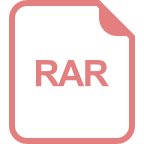
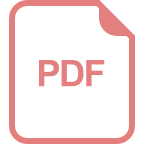
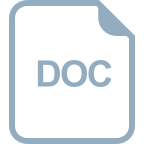
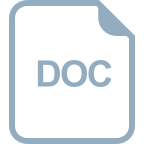
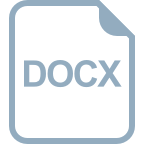
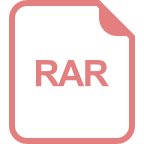
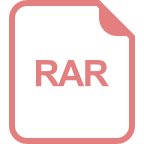
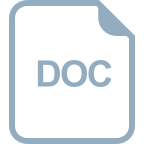
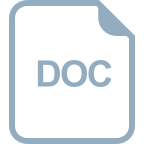
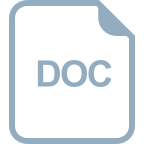


