用WPF写一段多视图切换,而且有良好的内存管理机制的代码
时间: 2024-05-13 11:13:28 浏览: 108
以下是一个基于WPF的多视图切换代码示例,它具有良好的内存管理机制:
MainWindow.xaml:
```xml
<Window x:Class="MultiViewSwitching.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Multi View Switching" Height="350" Width="525">
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<StackPanel Grid.Row="0" Orientation="Horizontal" HorizontalAlignment="Center">
<Button Content="View 1" Click="View1Button_Click"/>
<Button Content="View 2" Click="View2Button_Click"/>
<Button Content="View 3" Click="View3Button_Click"/>
</StackPanel>
<ContentControl Grid.Row="1" Content="{Binding CurrentView}"/>
</Grid>
</Window>
```
MainWindow.xaml.cs:
```csharp
using System.ComponentModel;
using System.Runtime.CompilerServices;
using System.Windows;
using MultiViewSwitching.Views;
namespace MultiViewSwitching
{
public partial class MainWindow : Window, INotifyPropertyChanged
{
private object _currentView;
public object CurrentView
{
get { return _currentView; }
set
{
if (_currentView != value)
{
_currentView = value;
OnPropertyChanged();
}
}
}
public MainWindow()
{
InitializeComponent();
// Set the initial view
CurrentView = new View1();
}
private void View1Button_Click(object sender, RoutedEventArgs e)
{
// Switch to view 1
CurrentView = new View1();
}
private void View2Button_Click(object sender, RoutedEventArgs e)
{
// Switch to view 2
CurrentView = new View2();
}
private void View3Button_Click(object sender, RoutedEventArgs e)
{
// Switch to view 3
CurrentView = new View3();
}
public event PropertyChangedEventHandler PropertyChanged;
protected virtual void OnPropertyChanged([CallerMemberName] string propertyName = null)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
}
```
View1.xaml:
```xml
<UserControl x:Class="MultiViewSwitching.Views.View1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="300">
<Grid>
<TextBlock Text="View 1"/>
</Grid>
</UserControl>
```
View2.xaml:
```xml
<UserControl x:Class="MultiViewSwitching.Views.View2"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="300">
<Grid>
<TextBlock Text="View 2"/>
</Grid>
</UserControl>
```
View3.xaml:
```xml
<UserControl x:Class="MultiViewSwitching.Views.View3"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="300">
<Grid>
<TextBlock Text="View 3"/>
</Grid>
</UserControl>
```
该示例使用ContentControl和数据绑定来实现多视图切换。每个视图都是一个用户控件,当用户点击按钮时,当前视图的Content属性将更改为新视图的实例。由于WPF具有内置的垃圾收集机制,因此不需要手动管理每个视图的内存。
阅读全文
相关推荐
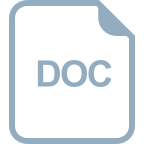
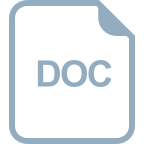
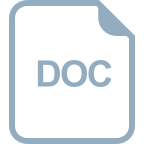
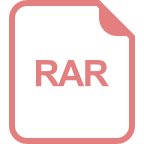
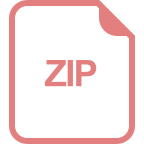
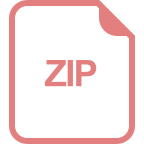
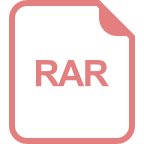
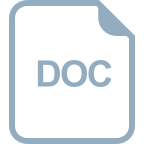
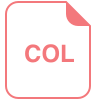
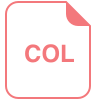
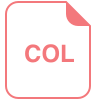
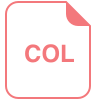
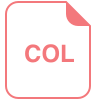
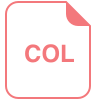
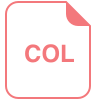
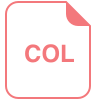
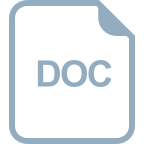
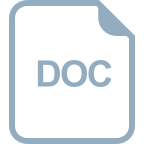
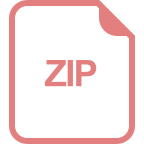
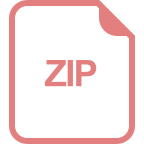