self.backbone = models.vgg19(pretrained=pretrained).features[:27]
时间: 2024-06-03 22:07:36 浏览: 10
This line of code initializes the backbone of the neural network using the VGG19 architecture. The "pretrained" parameter indicates whether to use the pre-trained weights or not. The backbone consists of the first 27 layers of the VGG19 network, which includes convolutional and pooling layers. This backbone is commonly used in object detection and image recognition tasks as it is known to perform well on these types of problems.
相关问题
self.backbone = resnet18(pretrained=True)
这行代码在PyTorch中创建了一个名为`backbone`的属性,并将其赋值为一个预训练的ResNet-18模型。下面是对这行代码的解释:
```python
self.backbone = resnet18(pretrained=True)
```
- `self`: 表示类的实例对象本身。
- `backbone`: 表示模型的骨干(backbone),通常用于提取图像特征。
- `resnet18`: 是一个PyTorch提供的预定义的ResNet-18模型,用于图像分类任务。ResNet-18由18个卷积层组成,包含了残差连接,可以有效地训练深层神经网络。
- `pretrained=True`: 表示使用在ImageNet数据集上预训练好的权重来初始化ResNet-18模型。预训练的权重可以帮助模型更快地收敛和更好地泛化。
通过将预训练的ResNet-18模型赋值给`self.backbone`属性,我们可以在模型中使用ResNet-18作为特征提取器,从而利用其强大的图像特征表示能力来增强我们的模型性能。
from collections import OrderedDict import torch import torch.nn.functional as F import torchvision from torch import nn import models.vgg_ as models class BackboneBase_VGG(nn.Module): def __init__(self, backbone: nn.Module, num_channels: int, name: str, return_interm_layers: bool): super().__init__() features = list(backbone.features.children()) if return_interm_layers: if name == 'vgg16_bn': self.body1 = nn.Sequential(*features[:13]) self.body2 = nn.Sequential(*features[13:23]) self.body3 = nn.Sequential(*features[23:33]) self.body4 = nn.Sequential(*features[33:43]) else: self.body1 = nn.Sequential(*features[:9]) self.body2 = nn.Sequential(*features[9:16]) self.body3 = nn.Sequential(*features[16:23]) self.body4 = nn.Sequential(*features[23:30]) else: if name == 'vgg16_bn': self.body = nn.Sequential(*features[:44]) # 16x down-sample elif name == 'vgg16': self.body = nn.Sequential(*features[:30]) # 16x down-sample self.num_channels = num_channels self.return_interm_layers = return_interm_layers def forward(self, tensor_list): out = [] if self.return_interm_layers: xs = tensor_list for _, layer in enumerate([self.body1, self.body2, self.body3, self.body4]): xs = layer(xs) out.append(xs) else: xs = self.body(tensor_list) out.append(xs) return out class Backbone_VGG(BackboneBase_VGG): """ResNet backbone with frozen BatchNorm.""" def __init__(self, name: str, return_interm_layers: bool): if name == 'vgg16_bn': backbone = models.vgg16_bn(pretrained=True) elif name == 'vgg16': backbone = models.vgg16(pretrained=True) num_channels = 256 super().__init__(backbone, num_channels, name, return_interm_layers) def build_backbone(args): backbone = Backbone_VGG(args.backbone, True) return backbone if __name__ == '__main__': Backbone_VGG('vgg16', True)
这段代码是一个基于VGG的神经网络模型的定义,主要用于特征提取。其中包括两个类:`BackboneBase_VGG`和`Backbone_VGG`以及一个函数`build_backbone`。
`BackboneBase_VGG`类是VGG的基础类,用于构建VGG神经网络模型。它的初始化函数接受四个参数:`backbone`,`num_channels`,`name`和`return_interm_layers`,其中`backbone`表示VGG的主干网络,`num_channels`表示输出的通道数,`name`表示VGG的名称,`return_interm_layers`表示是否返回中间层的输出。
`Backbone_VGG`类继承自`BackboneBase_VGG`类,主要用于构建一个冻结BatchNorm的VGG神经网络模型。它的初始化函数接受两个参数:`name`和`return_interm_layers`,其中`name`表示VGG的名称,`return_interm_layers`表示是否返回中间层的输出。这个类的主要作用是将`BackboneBase_VGG`类中的属性和方法进行实例化,然后通过调用父类的`__init__`方法进行初始化。
`build_backbone`函数是用于构建VGG神经网络模型的函数,它接受一个参数`args`,其中包含了VGG神经网络模型的一些参数。在这个函数中,会调用`Backbone_VGG`类来构建一个VGG神经网络模型,并将其返回。
最后,在`if __name__ == '__main__':`中,会创建一个`Backbone_VGG`类的实例对象,并传入参数`'vgg16'`和`True`进行初始化。
相关推荐
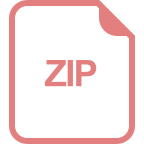
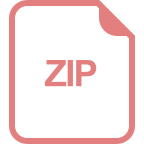












