js递归生成树形结构数据
时间: 2023-08-06 07:09:12 浏览: 122
好的,以下是一个简单的示例代码,用于将扁平的数据转换为树形结构数据:
```javascript
function generateTreeData(flatData, parentId = null) {
const treeData = [];
flatData.forEach(item => {
if (item.parentId === parentId) {
const children = generateTreeData(flatData, item.id);
if (children.length) {
item.children = children;
}
treeData.push(item);
}
});
return treeData;
}
```
以上代码接受两个参数:`flatData` 表示扁平的数据,`parentId` 表示当前节点的父节点ID。在函数内部,我们首先创建空的数组 `treeData`,然后遍历 `flatData` 中的每个元素。如果当前元素的 `parentId` 与指定的 `parentId` 相同,就将其添加到 `treeData` 中,并递归地调用 `generateTreeData` 函数来获取其子节点。最后返回 `treeData`。
要使用上述函数,只需要传入扁平的数据和根节点的ID,如下所示:
```javascript
const flatData = [
{ id: 1, name: '节点1', parentId: null },
{ id: 2, name: '节点2', parentId: 1 },
{ id: 3, name: '节点3', parentId: 1 },
{ id: 4, name: '节点4', parentId: 2 },
{ id: 5, name: '节点5', parentId: 2 },
{ id: 6, name: '节点6', parentId: 3 },
{ id: 7, name: '节点7', parentId: 3 },
];
const treeData = generateTreeData(flatData, null);
console.log(treeData);
```
以上代码将输出如下结果:
```javascript
[
{
"id": 1,
"name": "节点1",
"parentId": null,
"children": [
{
"id": 2,
"name": "节点2",
"parentId": 1,
"children": [
{
"id": 4,
"name": "节点4",
"parentId": 2,
"children": []
},
{
"id": 5,
"name": "节点5",
"parentId": 2,
"children": []
}
]
},
{
"id": 3,
"name": "节点3",
"parentId": 1,
"children": [
{
"id": 6,
"name": "节点6",
"parentId": 3,
"children": []
},
{
"id": 7,
"name": "节点7",
"parentId": 3,
"children": []
}
]
}
]
}
]
```
希望这个示例能够对你有所帮助!
阅读全文
相关推荐







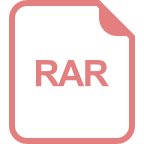

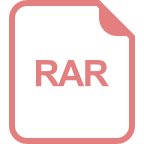






