c++邻接表 DFS算法判定有向无环图
时间: 2023-07-26 22:14:29 浏览: 178
邻接表表示的有向图可以通过深度优先搜索(DFS)算法来判定是否为有向无环图(DAG)。具体算法步骤如下:
1. 对每个节点维护三个状态:未访问(0)、正在访问(1)、已访问(2)。
2. 从任意一个未访问的节点开始深度优先遍历,每次遍历到一个节点时,将其状态设置为正在访问。
3. 对于当前节点的每个邻接节点,如果其状态是未访问,则对其进行递归访问。
4. 如果当前节点的任意邻接节点状态为正在访问,说明存在环,直接返回false。
5. 遍历完所有邻接节点后,将当前节点状态设置为已访问,返回true。
下面是具体的C++代码实现:
```c++
#include <iostream>
#include <vector>
using namespace std;
bool dfs(vector<vector<int>>& graph, vector<int>& visited, int cur) {
visited[cur] = 1; // 当前节点状态设置为正在访问
for (int next : graph[cur]) {
if (visited[next] == 0) { // 邻接节点未访问,递归访问
if (!dfs(graph, visited, next)) {
return false;
}
} else if (visited[next] == 1) { // 存在环,返回false
return false;
}
}
visited[cur] = 2; // 当前节点状态设置为已访问
return true;
}
bool isDAG(vector<vector<int>>& graph) {
int n = graph.size();
vector<int> visited(n, 0); // 初始化所有节点状态为未访问
for (int i = 0; i < n; i++) { // 从任意一个未访问的节点开始遍历
if (visited[i] == 0) {
if (!dfs(graph, visited, i)) {
return false;
}
}
}
return true;
}
int main() {
vector<vector<int>> graph = {{1, 2}, {3}, {3}, {4, 5}, {5}, {}};
if (isDAG(graph)) {
cout << "The graph is a DAG." << endl;
} else {
cout << "The graph is not a DAG." << endl;
}
return 0;
}
```
上面的代码中,`graph`是邻接表表示的有向图,`visited`是节点状态数组。`dfs`函数是深度优先遍历函数,`isDAG`函数则是判断有向图是否为DAG的函数。
阅读全文
相关推荐
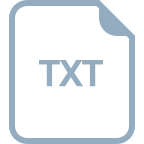
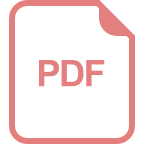

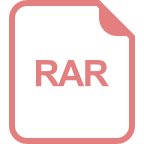
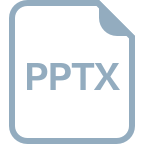
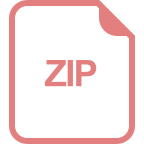
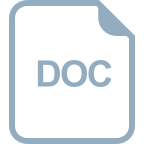
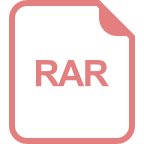
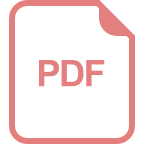
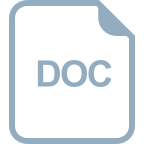
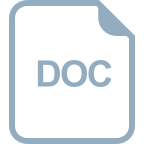
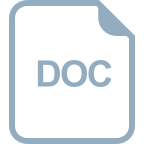
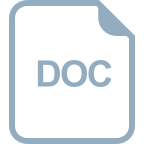
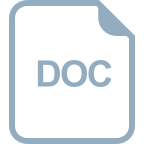
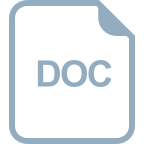
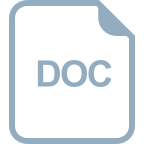
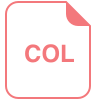