写程序可以自动读取每个子目录下的图片,每读一张图片生成一个列表 [图片x,标签],这里标签为图片所在目录的名字,如:0,1,2,3,... 将每次生成的列表作为一个元素加入总图片列表,最终把该总列表存入磁盘文件中。
时间: 2023-05-19 20:04:48 浏览: 68
可以使用Python中的os和PIL库来实现这个功能。具体实现步骤如下:
1. 使用os库遍历每个子目录,获取图片文件的路径。
2. 使用PIL库打开每个图片文件,获取图片数据和标签。
3. 将图片数据和标签组成一个元组,加入到一个列表中。
4. 遍历完所有图片后,将该列表加入到总图片列表中。
5. 将总图片列表存入磁盘文件中,可以使用pickle库来实现。
下面是示例代码:
```python
import os
from PIL import Image
import pickle
# 遍历目录,获取所有图片文件的路径
def get_image_paths(root_dir):
image_paths = []
for dirpath, dirnames, filenames in os.walk(root_dir):
for filename in filenames:
if filename.endswith('.jpg') or filename.endswith('.png'):
image_path = os.path.join(dirpath, filename)
image_paths.append(image_path)
return image_paths
# 读取图片数据和标签
def read_image(image_path):
with Image.open(image_path) as img:
image_data = img.tobytes()
label = os.path.basename(os.path.dirname(image_path))
return (image_data, label)
# 生成图片列表
def generate_image_list(root_dir):
image_list = []
image_paths = get_image_paths(root_dir)
for image_path in image_paths:
image_data, label = read_image(image_path)
image_list.append((image_data, label))
return image_list
# 存储图片列表到磁盘文件
def save_image_list(image_list, file_path):
with open(file_path, 'wb') as f:
pickle.dump(image_list, f)
# 测试代码
if __name__ == '__main__':
root_dir = 'image_dir'
image_list = generate_image_list(root_dir)
save_image_list(image_list, 'image_list.pkl')
```
其中,root_dir为图片所在的根目录,可以根据实际情况修改。运行该代码后,会在当前目录下生成一个名为image_list.pkl的文件,其中存储了所有图片的数据和标签。
相关推荐
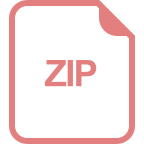















