python中如何在数组的指定位置插入另一个数组
时间: 2024-02-16 16:03:30 浏览: 26
可以使用Python中的切片(slice)操作来实现在数组的指定位置插入另一个数组。具体步骤如下:
1. 定义两个数组,一个是目标数组,一个是要插入的数组。
```python
target_array = [1, 2, 3, 4, 5]
insert_array = [6, 7, 8]
```
2. 使用切片操作将目标数组拆分成两部分,然后将要插入的数组插入到中间位置,最后再将两部分拼接起来。这里的中间位置指的是要插入的位置的前一个位置,也就是数组下标。
```python
insert_index = 2
target_array = target_array[:insert_index] + insert_array + target_array[insert_index:]
```
3. 最后得到的target_array就是在指定位置插入了另一个数组后的结果。
```python
print(target_array)
# 输出:[1, 2, 6, 7, 8, 3, 4, 5]
```
相关问题
python中如何在数组的指定位置插入另一个数组,但是新插入的数组不保留中括号
可以使用列表的切片和拼接操作来实现将一个数组插入到另一个数组的指定位置,同时去掉新插入数组的中括号。
具体操作步骤如下:
1. 定义两个数组,一个待插入的数组和一个目标数组。
```
insert_array = [1, 2, 3]
target_array = [4, 5, 6, 7, 8]
```
2. 使用切片操作将目标数组分为两部分,分别是插入位置之前的部分和插入位置之后的部分。
```
pos = 2
left = target_array[:pos]
right = target_array[pos:]
```
3. 将左部分和待插入数组拼接起来,然后再拼接右部分,得到插入新数组后的目标数组。
```
new_array = left + insert_array + right
```
4. 最后使用join函数将新数组转换为字符串并去掉中括号。
```
result = ", ".join(str(x) for x in new_array)
```
完整代码如下:
```python
insert_array = [1, 2, 3]
target_array = [4, 5, 6, 7, 8]
pos = 2
left = target_array[:pos]
right = target_array[pos:]
new_array = left + insert_array + right
result = ", ".join(str(x) for x in new_array)
print(result)
```
输出结果为:
```
4, 5, 1, 2, 3, 6, 7, 8
```
python中如何在数组的指定位置插入数字
在 Python 中,可以使用列表(List)来表示数组。要在指定位置插入数字,可以使用列表的 `insert()` 方法。该方法的语法如下:
```
list.insert(index, obj)
```
其中,`index` 表示要插入的位置,`obj` 表示要插入的对象(数字、字符串等)。例如,要在列表 `nums` 的第二个位置插入数字 `5`,可以使用以下代码:
```
nums = [1, 2, 3, 4]
nums.insert(1, 5)
print(nums)
```
输出结果为:`[1, 5, 2, 3, 4]`,可以看到数字 `5` 已经成功插入到了第二个位置。
相关推荐
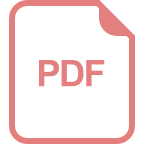













