node.js 正则表达式提取字符串
时间: 2023-09-16 18:09:15 浏览: 47
可以使用 JavaScript 中的正则表达式来提取字符串,在 Node.js 中同样适用。以下是一个简单的例子:
假设我们有一个字符串,格式为 "姓名:张三,年龄:20",我们想要提取出其中的姓名和年龄信息。可以使用正则表达式来实现:
```
const str = "姓名:张三,年龄:20";
const reg = /姓名:(.+?),年龄:(\d+)/;
const match = str.match(reg);
if (match) {
const name = match[1]; // "张三"
const age = match[2]; // "20"
}
```
这里我们定义了一个正则表达式 `reg`,它包含两个捕获组,分别用来匹配姓名和年龄信息。然后使用字符串的 `match` 方法来进行匹配,并得到一个数组 `match`,其中第一个元素为匹配到的整个字符串,后面的元素分别对应各个捕获组匹配到的内容。最后通过数组下标来获取需要的信息。
需要注意的是,使用正则表达式来提取字符串需要根据具体的需求来编写表达式,否则可能会得到错误的结果。
相关问题
node.js 正则表达式提取小数
可以使用正则表达式来提取小数。以下是一个示例代码:
```javascript
const str = '这是一个小数:3.1415926';
const regex = /(\d+\.\d+)/;
const match = str.match(regex);
if (match) {
console.log(match[1]); // 输出 3.1415926
} else {
console.log('没有匹配到小数');
}
```
正则表达式 `(\d+\.\d+)` 可以匹配一个或多个数字,后面跟着一个小数点和一个或多个数字。使用括号将匹配到的小数捕获到一个分组中,然后使用 `match` 方法匹配字符串并返回一个数组,数组中的第一个元素是整个匹配到的字符串,后面的元素是每个捕获分组的内容。因此,我们可以通过 `match[1]` 来获取到捕获到的小数。
node.js 正则表达式匹配小数
可以使用以下正则表达式匹配小数:
```javascript
/^\d+(\.\d+)?$/
```
该正则表达式的含义是:
- `^` 表示匹配字符串的开始位置。
- `\d+` 表示匹配一个或多个数字。
- `(\.\d+)?` 表示可选分组,匹配一个小数点和一个或多个数字。
- `$` 表示匹配字符串的结束位置。
示例代码:
```javascript
const decimalRegex = /^\d+(\.\d+)?$/;
console.log(decimalRegex.test('123')); // true
console.log(decimalRegex.test('123.45')); // true
console.log(decimalRegex.test('.45')); // false
console.log(decimalRegex.test('1.23.45')); // false
console.log(decimalRegex.test('12a3.45')); // false
```
相关推荐
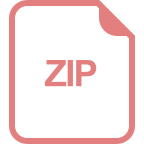
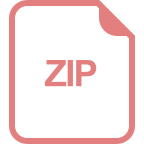
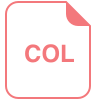
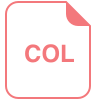
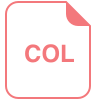
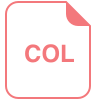








