用python实现将文件拷贝到指定目录
时间: 2024-05-07 17:16:21 浏览: 9
可以使用Python内置的shutil库来实现文件拷贝功能,示例代码如下:
```python
import shutil
# 拷贝文件到指定目录
src_file = 'path/to/source/file'
dst_dir = 'path/to/destination/directory'
shutil.copy(src_file, dst_dir)
```
注意,需要将`path/to/source/file`替换为待拷贝的文件路径,将`path/to/destination/directory`替换为目标目录路径。
相关问题
python2拷贝指定文件到指定目录下
以下是在Python 2.x中拷贝指定文件到指定目录的示例代码:
```python
import shutil
# 指定要拷贝的文件路径
src_file = "/path/to/source/file"
# 指定要拷贝到的目录路径
dest_dir = "/path/to/destination/directory"
# 使用shutil模块的copy函数进行拷贝操作
shutil.copy(src_file, dest_dir)
```
在上面的代码中,我们首先导入了Python标准库中的shutil模块,它提供了一些文件和目录操作的函数。然后,我们指定了要拷贝的文件路径和要拷贝到的目录路径,并使用shutil模块的copy函数进行拷贝操作。
如果要拷贝多个文件,可以使用os模块的listdir函数获取目录下的所有文件,然后使用循环进行拷贝操作:
```python
import os
import shutil
# 指定要拷贝的文件所在目录
src_dir = "/path/to/source/directory"
# 指定要拷贝到的目录路径
dest_dir = "/path/to/destination/directory"
# 获取目录下的所有文件
files = os.listdir(src_dir)
# 循环拷贝每个文件
for file in files:
src_file = os.path.join(src_dir, file)
shutil.copy(src_file, dest_dir)
```
在上面的代码中,我们首先指定了要拷贝的文件所在目录和要拷贝到的目录路径,然后使用os模块的listdir函数获取目录下的所有文件。接着,使用循环遍历每个文件,构造源文件路径,并使用shutil模块的copy函数进行拷贝操作。
python将文件复制到指定目录下
你可以使用shutil库中的copyfile函数来实现将文件复制到指定目录下的操作。首先,你需要导入shutil库和os库。然后,你可以使用os.listdir函数来获取指定目录下的所有文件列表。接下来,你可以使用一个循环来遍历文件列表,并使用shutil.copyfile函数将每个文件复制到目标目录下。下面是一个示例代码:
```python
import os
import shutil
source_dir = "D:\\notes\\python\\资料\\"
target_dir = "d:\\copy\\newname\\"
for file_name in os.listdir(source_dir):
if file_name.lower().endswith(".py"):
source_file = os.path.join(source_dir, file_name)
target_file = os.path.join(target_dir, file_name)
shutil.copyfile(source_file, target_file)
```
在这个示例中,我们首先定义了源目录和目标目录的路径。然后,使用os.listdir函数获取源目录下的所有文件列表。接下来,我们使用一个循环来遍历文件列表,并使用shutil.copyfile函数将每个以".py"结尾的文件复制到目标目录下。请注意,你需要根据你的实际情况修改源目录和目标目录的路径。
#### 引用[.reference_title]
- *1* [Python--将文件夹及其中的全部文件拷贝到指定路径下](https://blog.csdn.net/qq_33782655/article/details/127394877)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* [用python实现将文件拷贝到指定目录](https://blog.csdn.net/zd147896325/article/details/79870131)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
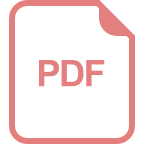
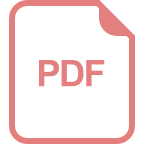












