python如何将csv文件中的行按第2列的时间先后顺序排序
时间: 2024-02-21 17:58:52 浏览: 66
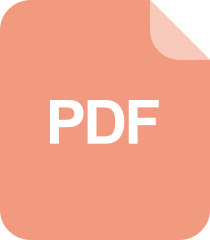
python实现对csv文件的读取和对第5列的排序
你可以使用 `csv` 模块和 `pandas` 库来按照 CSV 文件中的某一列排序。下面是使用 `csv` 模块实现的方法:
```python
import csv
# 读取 CSV 文件
with open('example.csv', 'r') as file:
reader = csv.reader(file)
# 按第2列的时间先后顺序排序并写入新的 CSV 文件
sorted_rows = sorted(reader, key=lambda row: row[1])
with open('new_example.csv', 'w', newline='') as new_file:
writer = csv.writer(new_file)
writer.writerows(sorted_rows)
```
这段代码会读取 `example.csv` 文件中的所有行,然后按照第2列的时间先后顺序排序,最后将排序后的行写入到 `new_example.csv` 文件中。在这里,我们使用了 `sorted()` 函数来排序,它的 `key` 参数指定了按照第2列来排序。注意,我们在 `open()` 函数中加入了参数 `newline=''`,这样可以避免写入 CSV 文件时出现空行。
如果你想使用 `pandas` 实现同样的功能,可以使用下面的代码:
```python
import pandas as pd
# 读取 CSV 文件
df = pd.read_csv('example.csv')
# 按第2列的时间先后顺序排序并将排序后的行写入新的 CSV 文件中
df = df.sort_values(by=['Column2'], ascending=True)
df.to_csv('new_example.csv', index=False)
```
这段代码会读取 `example.csv` 文件中的所有行,然后按照第2列的时间先后顺序排序,最后将排序后的行写入到 `new_example.csv` 文件中。在这里,我们使用了 `sort_values()` 方法来排序,它的 `by` 参数指定了按照第2列来排序,`ascending` 参数指定了升序排序。注意,我们在 `to_csv()` 函数中加入了参数 `index=False`,这样可以避免在写入 CSV 文件时出现行索引。
阅读全文
相关推荐



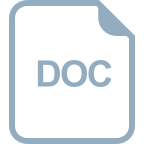






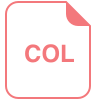






