JAVA RS485半双工通讯
时间: 2023-08-01 17:06:47 浏览: 50
Java可以通过串口通信来实现RS485半双工通讯。RS485是一种串行通信标准,可以实现在多个设备之间传输数据。在Java中,可以使用Java Communications API来实现串口通信。
首先需要安装Java Communications API,然后在Java代码中使用该API来打开串口。可以使用SerialPort类来打开串口,设置串口参数,并进行数据的读写操作。
下面是一个简单的Java代码示例,用于实现RS485半双工通讯:
```java
import gnu.io.CommPortIdentifier;
import gnu.io.SerialPort;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
public class RS485Test {
public static void main(String[] args) {
try {
// 获取串口
CommPortIdentifier portIdentifier = CommPortIdentifier.getPortIdentifier("COM1");
// 打开串口
SerialPort serialPort = (SerialPort) portIdentifier.open("RS485Test", 2000);
// 设置串口参数
serialPort.setSerialPortParams(9600, SerialPort.DATABITS_8, SerialPort.STOPBITS_1, SerialPort.PARITY_NONE);
serialPort.setFlowControlMode(SerialPort.FLOWCONTROL_NONE);
// 获取输入输出流
InputStream inputStream = serialPort.getInputStream();
OutputStream outputStream = serialPort.getOutputStream();
// 发送数据
byte[] sendData = new byte[] {0x01, 0x02, 0x03};
outputStream.write(sendData);
// 接收数据
byte[] receiveData = new byte[1024];
int len = 0;
while ((len = inputStream.read(receiveData)) > 0) {
System.out.println("Received data: " + new String(receiveData, 0, len));
}
// 关闭串口
serialPort.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
需要注意的是,RS485通讯需要在发送数据前先将控制线置为发送状态,在接收数据后再将控制线置为接收状态。此外,RS485通讯还需要注意数据的编码和解码方式,以及数据的校验等问题。
相关推荐
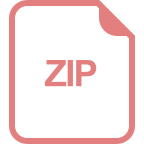












