python绘制迭代收敛图
时间: 2023-10-11 13:08:46 浏览: 345
下面是使用matplotlib库绘制迭代收敛图的示例代码:
```python
import matplotlib.pyplot as plt
# 定义迭代函数
def f(x):
return x**2 + 1
# 定义迭代次数
n = 100
# 定义初始点
x0 = 0.5
# 进行迭代
x = [x0]
for i in range(n):
x.append(f(x[-1]))
# 绘制收敛图
plt.plot(range(n+1), x, 'bo-')
plt.xlabel('Iteration')
plt.ylabel('x')
plt.title('Convergence Plot')
plt.show()
```
解释:
1. 定义迭代函数f(x),这里以 $f(x)=x^2+1$ 为例。
2. 定义迭代次数n,这里设为100。
3. 定义初始点x0,这里设为0.5。
4. 进行迭代,将每次迭代的结果存储在列表x中。
5. 绘制收敛图,横坐标为迭代次数,纵坐标为迭代得到的值x。使用蓝色圆点线条表示。添加横纵坐标标签和标题。最后调用show()方法显示图像。
执行上述代码,可以得到一个迭代收敛图,表示随着迭代次数的增加,初始点x0逐渐趋近于一个稳定的值。
相关问题
怎么绘制迭代收敛曲线图
要绘制迭代收敛曲线图,你可以使用Python的数据可视化库,如matplotlib或seaborn。下面是一个使用matplotlib绘制迭代收敛曲线图的简单示例:
```python
import matplotlib.pyplot as plt
# 模拟迭代过程,生成迭代收敛数据
iterations = range(1, 11)
convergence = [0.2, 0.4, 0.6, 0.8, 0.9, 0.95, 0.98, 0.99, 0.999, 1.0]
# 绘制迭代收敛曲线图
plt.plot(iterations, convergence, marker='o')
plt.xlabel('Iterations')
plt.ylabel('Convergence')
plt.title('Convergence Plot')
plt.grid(True)
plt.show()
```
在这个示例中,我们使用了一个包含10个迭代次数和对应收敛值的简单示例数据。使用`plt.plot`函数绘制曲线,并使用`plt.xlabel`、`plt.ylabel`和`plt.title`函数来为图表添加标签和标题。最后,使用`plt.grid(True)`函数添加网格线,并使用`plt.show()`函数显示图表。
你可以根据自己的数据和需求进行相应的修改和定制,来绘制适合你的迭代收敛曲线图。
如何在一张图中绘制两条迭代收敛曲线图
要在一张图中绘制两条迭代收敛曲线图,你可以使用matplotlib库的多次调用`plt.plot()`函数来绘制多条曲线。下面是一个示例:
```python
import matplotlib.pyplot as plt
# 模拟迭代过程,生成迭代收敛数据
iterations = range(1, 11)
convergence1 = [0.2, 0.4, 0.6, 0.8, 0.9, 0.95, 0.98, 0.99, 0.999, 1.0]
convergence2 = [0.3, 0.5, 0.7, 0.85, 0.92, 0.96, 0.98, 0.99, 0.999, 1.0]
# 绘制迭代收敛曲线图
plt.plot(iterations, convergence1, marker='o', label='Line 1')
plt.plot(iterations, convergence2, marker='x', label='Line 2')
plt.xlabel('Iterations')
plt.ylabel('Convergence')
plt.title('Convergence Plot')
plt.grid(True)
plt.legend()
plt.show()
```
在这个示例中,我们创建了两个迭代收敛数据列表:`convergence1`和`convergence2`。然后,我们通过两次调用`plt.plot()`函数来分别绘制这两条曲线。使用`label`参数来为每条曲线指定标签,在最后使用`plt.legend()`函数来显示图例。
你可以根据自己的需求和数据,添加更多的曲线和标签,来绘制多条迭代收敛曲线图。
阅读全文
相关推荐
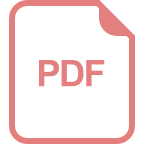
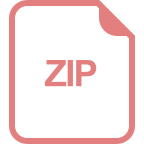
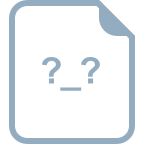

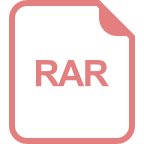
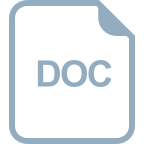
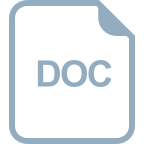
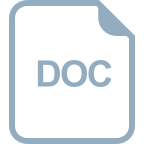
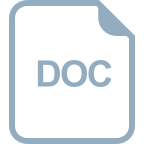
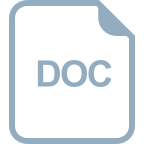
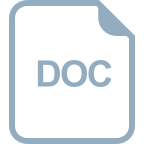


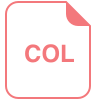


