用c++实现优先数调度算法,需要进程在CPU上的执行过程。要求每次进程调度时在屏幕上显示: 当前执行进程、就绪队列、等待队列。需要有注释,
时间: 2023-09-22 10:06:21 浏览: 178
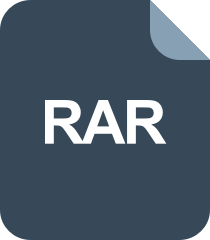
C++实现CPU调度算法先来先服务(FCFS),非抢占最短作业优先调度(SJF),优先级调度,时间片轮转调度(RR)并输出相应的平均等待时间和平均周转时间。

### 回答1:
以下是使用 C++ 实现优先数调度算法的示例代码,其中包括进程在 CPU 上的执行过程,并在屏幕上显示当前执行进程、就绪队列和等待队列。代码中有详细的注释说明。
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
// 进程结构体,包括进程编号、优先数、已执行时间和所需执行时间
struct Process {
int id;
int priority;
int executedTime;
int totalTime;
};
// 比较函数,按照优先数从小到大排序
bool compareProcess(const Process& p1, const Process& p2) {
return p1.priority < p2.priority;
}
int main() {
// 初始化进程队列
vector<Process> processList = {
{1, 2, 0, 10},
{2, 1, 0, 5},
{3, 3, 0, 8},
{4, 2, 0, 3},
{5, 1, 0, 6},
};
// 初始化就绪队列和等待队列
vector<Process> readyList;
vector<Process> waitList;
// 初始化当前执行进程为空指针
Process* currentProcess = nullptr;
// 执行调度算法,直到所有进程执行完毕
while (!processList.empty() || !readyList.empty() || currentProcess != nullptr) {
// 将已经完成执行的进程从当前执行进程中移除
if (currentProcess != nullptr && currentProcess->executedTime == currentProcess->totalTime) {
currentProcess = nullptr;
}
// 将已经完成执行的进程从就绪队列中移除
for (auto it = readyList.begin(); it != readyList.end();) {
if (it->executedTime == it->totalTime) {
it = readyList.erase(it);
} else {
++it;
}
}
// 将等待队列中等待时间已经超过 5 个时间片的进程移动到就绪队列中
for (auto it = waitList.begin(); it != waitList.end();) {
if (it->executedTime >= 5) {
readyList.push_back(*it);
it = waitList.erase(it);
} else {
++it;
}
}
// 将进程按照优先数从小到大排序
sort(processList.begin(), processList.end(), compareProcess);
// 将优先数最高的进程移动到就绪队列中
while (!processList.empty() && processList.back().priority >= 3) {
readyList.push_back(processList.back());
processList.pop_back();
}
// 如果当前执行进程为空指针,从就绪队列中选择优先数最高的进程作为当前执行进程
if (currentProcess == nullptr && !readyList.empty()) {
currentProcess = &(*min_element(readyList.begin(), readyList.end(), compareProcess));
}
// 输出当前执行进程、就绪队列和等待队列
cout << "当前执行进程:";
if (currentProcess != nullptr) {
cout << "进程" << currentProcess->id;
} else {
cout << "无";
}
cout << endl;
cout << "就绪队列:";
if (readyList.empty()) {
cout << "无";
} else {
for (const auto& process : readyList) {
cout << "进程" << process.id << "(" << process.priority << ") ";
}
}
cout << endl;
cout << "等待队列:";
if (waitList.empty()) {
cout << "无";
} else {
for (const auto& process : waitList) {
cout << "进程" << process.id << "(" << process.priority << ") ";
}
}
cout << endl;
// 如果当前执行进程不为空指针,执行一个时间片
if (currentProcess != nullptr) {
currentProcess->executedTime++;
// 如果进程执行完毕,将其移动到等待队列中
if (currentProcess->executedTime == currentProcess->totalTime) {
waitList.push_back(*currentProcess);
currentProcess = nullptr;
}
}
}
return 0;
}
```
在上述代码中,我们使用了 `vector` 容器来存储进程队列、就绪队列和等待队列,使用了结构体来表示进程的基本信息。在主循环中,我们首先移除已经完成执行的进程,然后移除已经完成执行的进程和等待时间已经超过 5 个时间片的进程,接着将进程按照优先数从小到大排序,并将优先数最高的进程移动到就绪队列中。如果当前执行进程为空指针,我们选择优先数最高的进程作为当前执行进程。最后,我们输出当前执行进程、就绪队列和等待队列,并根据当前执行进程是否为空指针来执行一个时间片。当进程队列、就绪队列和等待队列都为空,且当前执行进程为空指针时,主循环结束。
### 回答2:
优先数调度算法是一种根据进程优先级来进行进程调度的算法。在实现该算法前,我们首先需要定义进程的数据结构,包括进程号、进程优先级、进程状态等信息。
接下来我们可以使用C语言实现优先数调度算法,具体过程如下:
1. 定义进程结构体Process,包括进程号pid、进程优先级priority以及进程状态state。例如:
```
struct Process {
int pid;
int priority;
int state; // 0表示就绪状态,1表示执行状态,2表示等待状态
};
```
2. 定义就绪队列和等待队列,可以使用数组来实现。例如:
```
struct Process readyQueue[100]; // 就绪队列
struct Process waitingQueue[100]; // 等待队列
int readyCount = 0; // 就绪队列中进程的数量
int waitingCount = 0; // 等待队列中进程的数量
```
3. 实现进程调度函数schedule()。在此函数中,我们首先需要根据进程的优先级将进程排序,然后选择优先级最高的进程执行,同时将其状态设置为执行状态。例如:
```
void schedule() {
// 对就绪队列中的进程按照优先级进行排序
// 获取优先级最高的进程
struct Process current = readyQueue[0];
current.state = 1; // 设置状态为执行状态
// 输出当前执行进程、就绪队列、等待队列
printf("当前执行进程:%d\n", current.pid);
printf("就绪队列:");
for (int i = 0; i < readyCount; i++) {
printf("%d ", readyQueue[i].pid);
}
printf("\n");
printf("等待队列:");
for (int i = 0; i < waitingCount; i++) {
printf("%d ", waitingQueue[i].pid);
}
printf("\n");
}
```
4. 编写测试代码,模拟进程的创建、就绪和等待过程,并调用进程调度函数进行测试。
以上就是基于C语言实现优先数调度算法的大致思路和步骤,可以根据实际需求进行相应的修改和完善。
### 回答3:
优先数调度算法是一种根据进程优先级来进行调度的算法。下面是用C语言实现优先数调度算法,并在屏幕上显示当前执行进程、就绪队列和等待队列的代码,注释中详细解释了代码的功能。
```c
#include <stdio.h>
#define MAX_PROCESS 5
struct Process {
int priority; // 进程优先级
};
// 就绪队列
struct Process readyQueue[MAX_PROCESS];
// 等待队列
struct Process waitQueue[MAX_PROCESS];
// 当前执行进程
struct Process* currentProcess = NULL;
// 初始化进程队列
void initializeQueue(struct Process* queue) {
for (int i = 0; i < MAX_PROCESS; i++) {
queue[i].priority = 0;
}
}
// 打印当前执行进程、就绪队列和等待队列
void printState() {
printf("当前执行进程:%d\n", currentProcess != NULL ? currentProcess->priority : -1);
printf("就绪队列:");
for (int i = 0; i < MAX_PROCESS; i++) {
printf("%d ", readyQueue[i].priority);
}
printf("\n等待队列:");
for (int i = 0; i < MAX_PROCESS; i++) {
printf("%d ", waitQueue[i].priority);
}
printf("\n\n");
}
// 优先数调度算法
void priorityScheduling() {
// 首先找到就绪队列中优先级最高的进程
int maxPriority = -1;
int maxIndex = -1;
for (int i = 0; i < MAX_PROCESS; i++) {
if (readyQueue[i].priority > maxPriority) {
maxPriority = readyQueue[i].priority;
maxIndex = i;
}
}
// 执行最高优先级的进程
currentProcess = &readyQueue[maxIndex];
// 将其他就绪队列中的进程向前移动
for (int i = maxIndex; i < MAX_PROCESS - 1; i++) {
readyQueue[i] = readyQueue[i + 1];
}
// 将最后一个进程从就绪队列中删除
readyQueue[MAX_PROCESS - 1].priority = 0;
// 打印当前状态
printState();
}
int main() {
// 初始化就绪队列和等待队列
initializeQueue(readyQueue);
initializeQueue(waitQueue);
// 添加进程到就绪队列
readyQueue[0].priority = 3;
readyQueue[1].priority = 1;
readyQueue[2].priority = 2;
readyQueue[3].priority = 5;
readyQueue[4].priority = 4;
// 调度进程
while (1) {
priorityScheduling();
// 执行完毕后将当前进程添加到等待队列
int waitIndex = 0;
while (waitQueue[waitIndex].priority != 0) {
waitIndex++;
}
waitQueue[waitIndex] = *currentProcess;
currentProcess = NULL;
}
return 0;
}
```
以上代码实现了一个简单的优先数调度算法,根据进程优先级来决定进程的执行顺序。输出中会显示当前执行进程、就绪队列和等待队列的情况,在每次进程调度时会更新并显示这些信息。请根据实际需求进行修改和扩展。
阅读全文
相关推荐
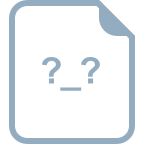
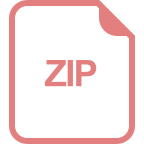
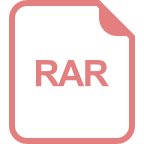
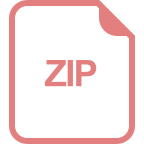
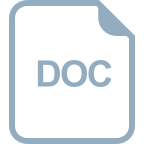
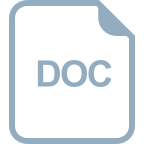
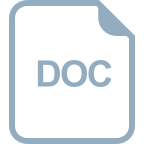
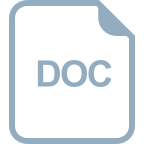







