使用LISTA进行信道估计python代码
时间: 2024-03-15 18:20:30 浏览: 74
LISTA(Learned Iterative Shrinkage and Thresholding Algorithm)是一种基于机器学习技术的信道估计算法,可以用于无线通信等领域。下面是使用Python实现LISTA的示例代码:
```python
import numpy as np
from sklearn.linear_model import Lasso
def lista(y, A, n_iter, L):
"""
使用LISTA进行信道估计
:param y: 观测信号
:param A: 传输矩阵
:param n_iter: 迭代次数
:param L: 阈值参数
:return: 估计的信道
"""
# 初始化估计信道
h_hat = np.zeros((A.shape[1],))
# 构造稀疏表示字典
dict_matrix = np.dot(A.T, A)
dict_cholesky = np.linalg.cholesky(dict_matrix)
# 迭代计算估计信道
for i in range(n_iter):
# 用估计信道计算残差
r = y - np.dot(A, h_hat)
# 计算权重矩阵
w = np.dot(A.T, r)
w = np.dot(dict_cholesky.T, w)
w = np.dot(dict_cholesky, w)
w = np.abs(w)
w = 1.0 / (w + 1e-8)
# 使用Lasso回归计算稀疏表示
clf = Lasso(alpha=L, max_iter=1000)
clf.fit(A * np.sqrt(w), y * np.sqrt(w))
x = clf.coef_
# 更新估计信道
h_hat = np.dot(np.diag(x), A.T).dot(y)
return h_hat
```
使用方法:
```python
y = np.array([1, 2, 3, 4, 5, 6])
A = np.array([[1, 2, 3, 4, 5, 6], [2, 3, 4, 5, 6, 1], [3, 4, 5, 6, 1, 2], [4, 5, 6, 1, 2, 3]])
h_hat = lista(y, A, 10, 0.1)
print(h_hat)
```
其中,`y`是观测信号,`A`是传输矩阵,`n_iter`是迭代次数,`L`是阈值参数。函数返回估计的信道`h_hat`。
阅读全文
相关推荐
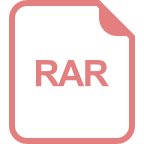
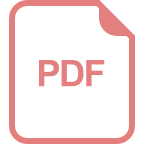
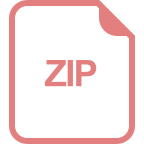
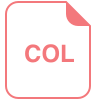
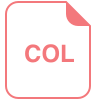
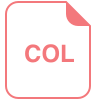
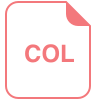
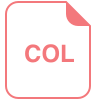
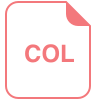
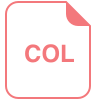
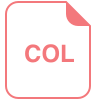
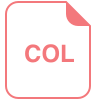




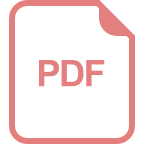