用Python代码实现从‘202209191727’中提取出前8个字符‘20220919’
时间: 2024-05-01 09:18:07 浏览: 44
好的,以下是实现代码:
```python
s = '202209191727'
result = s[:8]
print(result)
```
输出结果为:'20220919'
需要注意的是,可以使用字符串的切片功能来实现从一个字符串中提取出需要的部分。在这个例子中,用 s[:8] 表示提取字符串 s 的前8个字符。
相关问题
将两个字符串中的数字提取出来作差,用Python代码实现
可以使用正则表达式将字符串中的数字提取出来,然后进行计算。下面是一个简单的Python代码实现:
```python
import re
s1 = "hello 123 world"
s2 = "hello 456 world"
# 使用正则表达式提取数字
num1 = int(re.findall("\d+", s1)[0])
num2 = int(re.findall("\d+", s2)[0])
# 计算差值
diff = num1 - num2
print(diff) # 输出结果为 -333
```
以上代码中,使用正则表达式 `\d+` 匹配字符串中的数字,并使用 `re.findall` 方法返回一个包含所有匹配项的列表。由于本例中只有一个数字,所以直接使用 `[0]` 取出列表中的第一个元素。最后将两个数字相减得到差值。
使用Python实现提取文本中的特定字符串的代码实现
以下是使用Python实现提取文本中的特定字符串的代码实现:
```python
import re
text = "Hello, my name is John. My email is john@example.com. Please contact me at your earliest convenience."
# 使用正则表达式提取邮箱
regex = r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b'
emails = re.findall(regex, text)
print(emails)
# Output: ['john@example.com']
```
这段代码可以从给定的文本中提取出所有符合邮箱格式的字符串,输出为一个包含所有邮箱的列表。
阅读全文
相关推荐
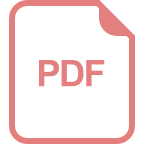
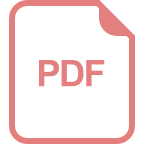
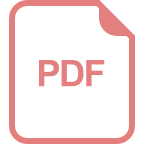













