用python推理tensorrt模型的代码
时间: 2023-08-29 13:10:44 浏览: 122
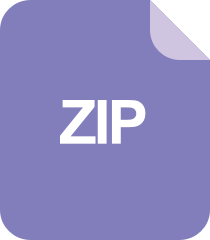
基于python部署fastsam的tensorrt模型源码.zip
以下是一个基本的Python代码示例,用于使用TensorRT推理一个经过优化的模型:
```python
import tensorrt as trt
import pycuda.autoinit
import pycuda.driver as cuda
import numpy as np
# Load the serialized engine from file
TRT_LOGGER = trt.Logger(trt.Logger.WARNING)
with open("model.engine", "rb") as f:
engine_data = f.read()
# Deserialize the engine
runtime = trt.Runtime(TRT_LOGGER)
engine = runtime.deserialize_cuda_engine(engine_data)
# Allocate input and output memory buffers
input_shape = (1, 3, 224, 224)
output_shape = (1, 1000)
input_host = cuda.pagelocked_empty(np.prod(input_shape), dtype=np.float32)
output_host = cuda.pagelocked_empty(np.prod(output_shape), dtype=np.float32)
input_device = cuda.mem_alloc(input_host.nbytes)
output_device = cuda.mem_alloc(output_host.nbytes)
# Create a CUDA stream for device memory operations
stream = cuda.Stream()
# Create an execution context from the deserialized engine
context = engine.create_execution_context()
# Copy input data to device memory
cuda.memcpy_htod_async(input_device, input_host, stream)
# Execute the inference engine
context.execute_async_v2(bindings=[int(input_device), int(output_device)], stream_handle=stream.handle)
# Copy output data from device memory to host memory
cuda.memcpy_dtoh_async(output_host, output_device, stream)
# Synchronize the stream to ensure the computation is complete
stream.synchronize()
# Print the output tensor
print(output_host)
```
在这个示例中,我们从文件中加载序列化的TensorRT引擎,并使用它来创建一个执行上下文。然后,我们使用PyCUDA来分配输入和输出内存缓冲区,并使用CUDA流将输入数据从主机内存复制到设备内存。接下来,我们执行推理引擎,并使用CUDA流将输出数据从设备内存复制回主机内存。最后,我们打印输出张量以查看结果。
请注意,这只是一个基本的示例,可以根据您的具体要求进行修改和扩展。
阅读全文
相关推荐
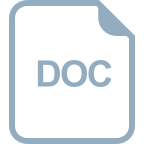
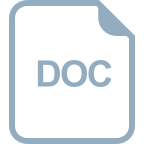


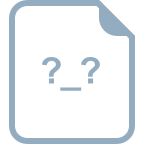

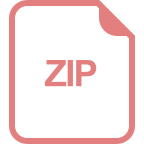
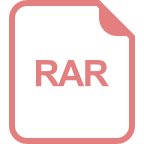
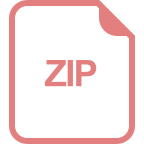
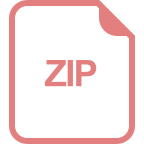
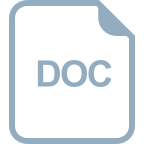






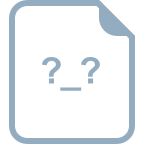