python 推理tensorrt模型
时间: 2023-08-29 19:10:56 浏览: 158
要在 Python 中推理 TensorRT 模型,您需要使用 TensorRT Python API。TensorRT Python API 是一个 Python 包,它提供了一组用于加载、优化和推理 TensorRT 模型的函数和类。
下面是一些步骤,帮助您在 Python 中推理 TensorRT 模型:
1. 安装 TensorRT Python API:您需要从 NVIDIA 的官方网站下载和安装 TensorRT Python API。请确保您的系统满足 TensorRT Python API 的要求。
2. 加载和优化 TensorRT 模型:使用 TensorRT Python API 的函数和类来加载和优化 TensorRT 模型。您可以使用 TensorRT Python API 的 Builder 类来构建和优化 TensorRT 引擎。
3. 推理 TensorRT 模型:使用 TensorRT Python API 的函数和类来推理 TensorRT 模型。您可以使用 TensorRT Python API 的 Engine 类来执行推理操作。
4. 处理输出:您可以使用 Python 来处理 TensorRT 模型的输出。您可以将 TensorRT 模型的输出转换为 NumPy 数组,并使用 NumPy 函数对其进行处理。
下面是一个简单的示例,展示如何在 Python 中推理 TensorRT 模型:
```python
import tensorrt as trt
import numpy as np
# 加载 TensorRT 模型
TRT_LOGGER = trt.Logger(trt.Logger.WARNING)
with open("model.trt", "rb") as f:
engine = trt.Runtime(TRT_LOGGER).deserialize_cuda_engine(f.read())
# 创建 TensorRT 推理上下文
context = engine.create_execution_context()
# 准备输入数据
input_data = np.random.normal(size=(1, 3, 224, 224)).astype(np.float32)
# 执行推理操作
bindings = [None] * engine.num_bindings
inputs_idx = [engine.get_binding_index(name) for name in input_names]
outputs_idx = [engine.get_binding_index(name) for name in output_names]
bindings[inputs_idx[0]] = input_data
output_data = np.empty(shape=engine.get_binding_shape(outputs_idx[0]), dtype=np.float32)
bindings[outputs_idx[0]] = output_data
context.execute_v2(bindings)
# 处理输出数据
output_data = output_data.reshape(1, -1)
output_data = np.argmax(output_data, axis=1)
print(output_data)
```
在这个示例中,我们首先加载了一个 TensorRT 模型,并创建了一个 TensorRT 推理上下文。然后,我们准备了输入数据,并使用 TensorRT 推理上下文执行了推理操作。最后,我们将输出数据转换为 NumPy 数组,并使用 NumPy 函数对其进行处理。
阅读全文
相关推荐
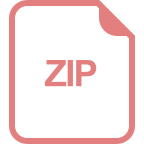


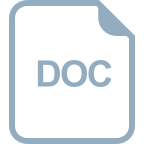

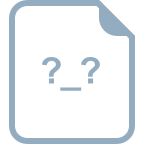
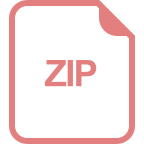
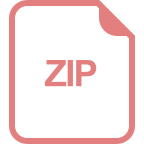
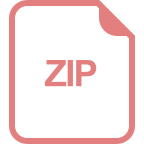
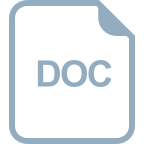
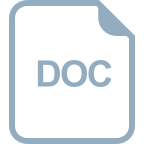
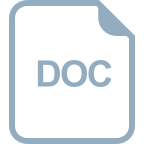




