网页抓取广东省湛江市近15天天气高低温度,将数据处理结果保存进入mysql,然后用pyecharts处理结果可视化,最后用flask联通前后端,额外添加首页界面(bootstrap),登录界面
时间: 2023-09-27 07:05:32 浏览: 42
好的,这是一个比较完整的项目,需要分步骤进行。首先需要安装一些依赖库,包括 requests、beautifulsoup4、pymysql、pyecharts、flask、bootstrap。
1. 网页抓取
利用requests库获取网页内容,再使用beautifulsoup4库进行解析,获取需要的数据。
```python
import requests
from bs4 import BeautifulSoup
url = 'http://www.weather.com.cn/weather15d/101281001.shtml'
res = requests.get(url)
res.encoding = 'utf-8'
soup = BeautifulSoup(res.text, 'html.parser')
data = []
for i in range(15):
d = {}
d['date'] = soup.select('.t15d')[0].select('li')[i].select('.day')[0].text.strip()
d['week'] = soup.select('.t15d')[0].select('li')[i].select('.week')[0].text.strip()
d['high'] = soup.select('.t15d')[0].select('li')[i].select('.tem')[0].select('span')[0].text.strip()
d['low'] = soup.select('.t15d')[0].select('li')[i].select('.tem')[0].select('i')[0].text.strip()
data.append(d)
```
2. 数据处理与存储
将抓取到的数据进行处理,转换成需要的格式,然后使用pymysql库连接数据库并插入数据。
```python
import pymysql
conn = pymysql.connect(host='localhost', user='root', password='password', database='weather')
cursor = conn.cursor()
for d in data:
date = d['date']
week = d['week']
high = int(d['high'].replace('℃', ''))
low = int(d['low'].replace('℃', ''))
sql = f"INSERT INTO weather (date, week, high, low) VALUES ('{date}', '{week}', {high}, {low})"
cursor.execute(sql)
conn.commit()
conn.close()
```
3. 数据可视化
使用pyecharts库将数据进行可视化处理,生成一个折线图。
```python
from pyecharts.charts import Line
from pyecharts import options as opts
from pyecharts.globals import ThemeType
conn = pymysql.connect(host='localhost', user='root', password='password', database='weather')
cursor = conn.cursor()
cursor.execute("SELECT * FROM weather")
results = cursor.fetchall()
date_list = [result[1] for result in results]
high_list = [result[3] for result in results]
low_list = [result[4] for result in results]
line = Line(init_opts=opts.InitOpts(theme=ThemeType.LIGHT))
line.add_xaxis(date_list)
line.add_yaxis('最高温度', high_list)
line.add_yaxis('最低温度', low_list)
line.set_global_opts(title_opts=opts.TitleOpts(title='湛江市近15天天气数据'))
line.render('weather.html')
```
4. Flask搭建
使用Flask框架搭建一个简单的web应用,将数据可视化结果作为一个页面输出。
```python
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
@app.route('/weather')
def weather():
return render_template('weather.html')
if __name__ == '__main__':
app.run()
```
5. 额外添加首页界面和登录界面
使用bootstrap模板制作一个简单的首页界面和登录界面,可以参考bootstrap文档中的示例代码进行制作。在Flask应用中设置相应的路由,将两个页面进行输出。
```python
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
@app.route('/login')
def login():
return render_template('login.html')
@app.route('/weather')
def weather():
return render_template('weather.html')
if __name__ == '__main__':
app.run()
```
以上是整个项目的基本实现流程,需要注意的是,需要在本地安装好mysql数据库,并且创建一个名为weather的数据库,在其中创建一张名为weather的表,表中包含四个字段,分别为id、date、week、high、low。另外,需要在Flask应用中设置好数据库的连接信息。
相关推荐
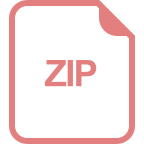
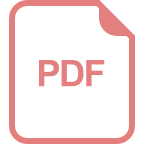
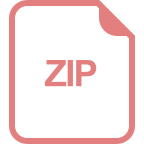














