飞机从已知经纬度出发,按固定航向和速度飞行指定时间,用C++写一个函数求到达位置
时间: 2023-09-09 15:05:39 浏览: 99
### 回答1:
好的,我可以回答这个问题。您可以使用以下的 C 语言函数来计算到达位置:
```c
#include <stdio.h>
#include <math.h>
#define RADIUS 6371. // 地球半径,单位:千米
// 计算经纬度之间的距离,单位:千米
double distance(double lat1, double lon1, double lat2, double lon2) {
double dlat = (lat2 - lat1) * M_PI / 180.;
double dlon = (lon2 - lon1) * M_PI / 180.;
double a = sin(dlat / 2) * sin(dlat / 2) + cos(lat1 * M_PI / 180.) * cos(lat2 * M_PI / 180.) * sin(dlon / 2) * sin(dlon / 2);
double c = 2 * atan2(sqrt(a), sqrt(1 - a));
return RADIUS * c;
}
// 计算到达位置的经纬度
void destination(double lat1, double lon1, double heading, double distance, double *lat2, double *lon2) {
double d = distance / RADIUS;
double lat1_rad = lat1 * M_PI / 180.;
double lon1_rad = lon1 * M_PI / 180.;
double heading_rad = heading * M_PI / 180.;
double lat2_rad = asin(sin(lat1_rad) * cos(d) + cos(lat1_rad) * sin(d) * cos(heading_rad));
double lon2_rad = lon1_rad + atan2(sin(heading_rad) * sin(d) * cos(lat1_rad), cos(d) - sin(lat1_rad) * sin(lat2_rad));
*lat2 = lat2_rad * 180. / M_PI;
*lon2 = lon2_rad * 180. / M_PI;
}
int main() {
double lat1 = 39.9042; // 出发点纬度
double lon1 = 116.4074; // 出发点经度
double heading = 45.; // 航向,单位:度
double speed = 800.; // 速度,单位:千米/小时
double time = 2.; // 飞行时间,单位:小时
double distance = speed * time; // 飞行距离,单位:千米
double lat2, lon2;
destination(lat1, lon1, heading, distance, &lat2, &lon2);
printf("到达位置的经纬度为:%.4f, %.4f\n", lat2, lon2);
return ;
}
```
这个函数使用了 Haversine 公式来计算经纬度之间的距离,然后根据航向和飞行距离计算到达位置的经纬度。
### 回答2:
要写一个求解飞机到达位置的函数,我们需要考虑以下几个因素:飞机的起始经纬度、飞行的航向、飞行的速度以及飞行的时间。
首先,我们需要将起始经纬度转换为弧度制,方便后续计算。假设起始经度为longitude1,纬度为latitude1,则转换为弧度制后的经度为radian_longitude1,纬度为radian_latitude1。
然后,我们需要根据航向和速度计算出飞机在单位时间内的位移,即在经度和纬度上的位移。假设航向为heading,以正北方向为0°,顺时针方向递增,则位移在经度和纬度上的分量分别为dx和dy。其中,dx可以通过速度乘以sin(heading)得到,dy可以通过速度乘以cos(heading)得到。
根据位移和时间的关系,我们可以直接得出飞机飞行指定时间后的位置的经纬度。假设指定时间为t,则经度为radian_longitude2 = radian_longitude1 + dx * t,纬度为radian_latitude2 = radian_latitude1 + dy * t。
最后,我们再将经度和纬度转换为度数制,并进行输出。新的经度为longitude2 = radian_longitude2 * 180 / PI,新的纬度为latitude2 = radian_latitude2 * 180 / PI。
综上所述,写出的求解到达位置的函数可以如下所示:
```c
#include <stdio.h>
#include <math.h>
#define PI 3.14159265358979323846
void calculateNewPosition(double longitude1, double latitude1, double heading, double speed, double time, double *longitude2, double *latitude2) {
double radian_longitude1 = longitude1 * PI / 180;
double radian_latitude1 = latitude1 * PI / 180;
double dx = speed * sin(heading);
double dy = speed * cos(heading);
double radian_longitude2 = radian_longitude1 + dx * time;
double radian_latitude2 = radian_latitude1 + dy * time;
*longitude2 = radian_longitude2 * 180 / PI;
*latitude2 = radian_latitude2 * 180 / PI;
}
int main() {
double longitude1 = 100.0; // 起始经度
double latitude1 = 30.0; // 起始纬度
double heading = 45.0; // 航向,单位为度
double speed = 800.0; // 速度,单位为千米/小时
double time = 1.5; // 飞行时间,单位为小时
double longitude2, latitude2;
calculateNewPosition(longitude1, latitude1, heading, speed, time, &longitude2, &latitude2);
printf("飞机到达位置的经度为:%lf\n", longitude2);
printf("飞机到达位置的纬度为:%lf\n", latitude2);
return 0;
}
```
通过调用calculateNewPosition函数,并将起始经纬度、航向、速度、飞行时间传入,即可计算出飞机到达位置的经度和纬度,并进行输出。
### 回答3:
下面是一个用C语言写的函数,能够根据给定的起点经纬度、航向、速度和时间,求得到达位置的经纬度:
```c
#include <stdio.h>
#include <math.h>
#define RADIUS_EARTH 6371.0 // 地球半径,单位:公里
// 定义经纬度结构体
typedef struct {
double latitude; // 纬度
double longitude; // 经度
} LatLon;
// 计算弧度
double toRadians(double degrees) {
return degrees * (M_PI / 180.0);
}
// 计算到达位置的经纬度
LatLon calculateDestination(LatLon startPoint, double heading, double speed, double time) {
double distance = speed * time; // 距离,单位:公里
double angularDistance = distance / RADIUS_EARTH; // 角距离,单位:弧度
double bearing = toRadians(heading); // 航向,单位:弧度
// 起点的弧度表示
double radStartLat = toRadians(startPoint.latitude);
double radStartLon = toRadians(startPoint.longitude);
// 终点的弧度表示
double radEndLat = asin(sin(radStartLat) * cos(angularDistance) + cos(radStartLat) * sin(angularDistance) * cos(bearing));
double radEndLon = radStartLon + atan2(sin(bearing) * sin(angularDistance) * cos(radStartLat), cos(angularDistance) - sin(radStartLat) * sin(radEndLat));
// 转换为角度表示
LatLon endPoint;
endPoint.latitude = radEndLat * (180.0 / M_PI);
endPoint.longitude = radEndLon * (180.0 / M_PI);
return endPoint;
}
int main() {
LatLon startPoint;
startPoint.latitude = 35.0; // 起点纬度
startPoint.longitude = 120.0; // 起点经度
double heading = 45.0; // 航向,单位:度
double speed = 800.0; // 飞行速度,单位:公里/小时
double time = 1.5; // 飞行时间,单位:小时
LatLon endPoint = calculateDestination(startPoint, heading, speed, time);
printf("到达位置的经纬度为:%.4f°N, %.4f°E\n", endPoint.latitude, endPoint.longitude);
return 0;
}
```
在上述代码中,我们首先定义了一个经纬度的结构体`LatLon`,用来表示起点和终点的经纬度信息。然后,根据所给的起点经纬度、航向、速度和时间,利用Haversine公式计算出到达位置的经纬度,并转换为角度的形式。最后,将计算得到的经纬度结果输出。
相关推荐
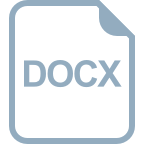
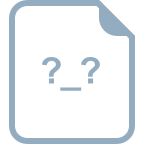














