1. 编一个程序,测量每一种基本数据类型所占字节数。
时间: 2024-09-23 18:13:47 浏览: 62
编写一个简单的程序来测量基本数据类型所占用的字节数可以使用各种编程语言,下面是一个使用Python的例子,因为它相对直观且易于理解:
```python
# Python 3
def measure_data_type_sizes():
types_and_sizes = {
"int8": sizeof(short),
"int16": sizeof(int),
"int32": sizeof(long),
"int64": sizeof(long long),
"float": sizeof(float),
"double": sizeof(double),
"char": sizeof(char),
"bool": sizeof(bool)
}
for data_type, size in types_and_sizes.items():
print(f"{data_type}: {size} bytes")
measure_data_type_sizes()
```
这个程序通过`sizeof()`函数获取每个数据类型的大小(以字节为单位),然后打印出来。请注意,实际的结果可能会因不同的操作系统和架构(如32位或64位系统)有所不同。
如果你想在其他语言(如C++、Java等)中做类似的事情,你可以查阅相应的文档找到对应的数据类型大小。
相关问题
用c语言编一个程序测量每一种基本数据类型所占字节数
在 C 语言中,你可以创建一个简单的程序来测量基本数据类型的大小,包括整型、浮点型、字符型等。以下是一个简单的示例:
```c
#include <stdio.h>
int main() {
// 整型(int)
int int_size;
printf("Size of int: %d bytes\n", sizeof(int_size));
// 浮点型(float, double)
float float_size;
printf("Size of float: %d bytes\n", sizeof(float_size));
double double_size;
printf("Size of double: %d bytes\n", sizeof(double_size));
// 字符型(char)
char char_size;
printf("Size of char: %d bytes\n", sizeof(char_size));
// 结构体类型(可以包含基本类型)
struct ExampleStruct {
int value;
float fvalue;
char cvalue;
} struct_example;
printf("Size of a structure with basic types: %d bytes\n", sizeof(struct_example));
return 0;
}
```
当你运行这个程序时,它会打印出每种数据类型占用的字节数。注意,在实际应用中,这些值可能会因不同的系统架构(如32位或64位)而有所不同。
编一个程序,测量每一种基本数据类型所占字节数
编写一个简单的Java程序可以测量各种基本数据类型的大小(字节数)。这里是一个示例:
```java
public class SizeMeasurement {
public static void main(String[] args) {
// 测量整数(int)
int integerSize = Integer.SIZE / 8;
System.out.println("Integer size in bytes: " + integerSize);
// 测量浮点数(float)
float floatValue = Float.SIZE / 8;
System.out.println("Float size in bytes: " + floatValue);
// 测量双精度浮点数(double)
double doubleValue = Double.SIZE / 8;
System.out.println("Double size in bytes: " + doubleValue);
// 测量字符(char)
char charSize = Character.SIZE / 8;
System.out.println("Character size in bytes: " + charSize);
// 测量布尔(boolean)
boolean booleanSize = Boolean.SIZE / 8;
System.out.println("Boolean size in bytes: " + booleanSize);
// 测量短整型(short)
short shortSize = Short.SIZE / 8;
System.out.println("Short size in bytes: " + shortSize);
// 测量长整型(long)
long longSize = Long.SIZE / 8;
System.out.println("Long size in bytes: " + longSize);
}
}
```
运行这个程序会打印出每个基本数据类型的字节大小。请注意,在不同的平台和架构上,可能会有细微差异,因为这是由系统的位宽和内存对齐规则决定的。
阅读全文
相关推荐
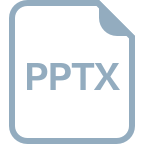
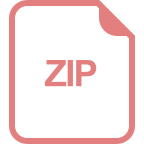
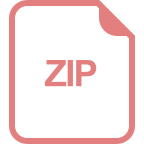
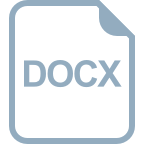
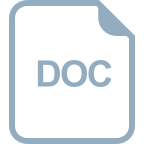
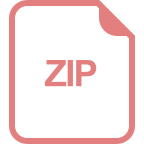
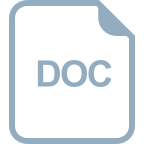
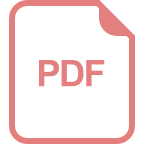
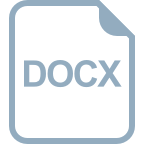
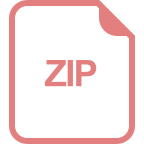
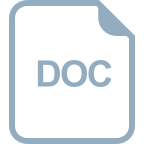
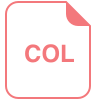
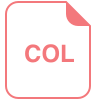
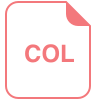
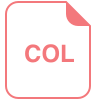
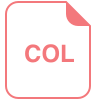
