List<Long>去重
时间: 2024-05-21 13:11:34 浏览: 213
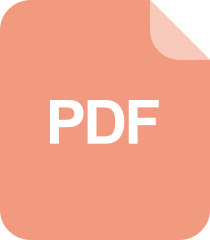
Java实现的数组去重与排序操作详解
可以使用Java 8的stream和distinct方法来实现List<Long>去重,示例代码如下:
List<Long> list = Arrays.asList(1L, 2L, 3L, 2L, 4L);
List<Long> distinctList = list.stream().distinct().collect(Collectors.toList());
System.out.println(distinctList); // Output: [1, 2, 3, 4]
阅读全文
相关推荐
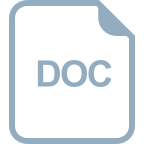
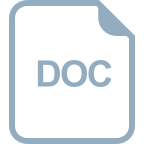















