写一段C语言代码,包含结构体
时间: 2023-09-15 12:05:42 浏览: 59
### 回答1:
#include <stdio.h>
struct student{
int age;
float score;
};int main(){
struct student s1;
s1.age = 18;
s1.score = 95.5;
printf("学生的年龄是:%d, 成绩是:%.2f\n", s1.age, s1.score);
return 0;
}
### 回答2:
#include <stdio.h>
#include <string.h>
// 定义结构体学生
struct Student {
char name[20];
int age;
float score;
};
int main() {
struct Student std1, std2;
// 输入第一个学生信息
printf("请输入第一个学生的姓名:");
scanf("%s", std1.name);
printf("请输入第一个学生的年龄:");
scanf("%d", &std1.age);
printf("请输入第一个学生的成绩:");
scanf("%f", &std1.score);
// 输入第二个学生信息
printf("请输入第二个学生的姓名:");
scanf("%s", std2.name);
printf("请输入第二个学生的年龄:");
scanf("%d", &std2.age);
printf("请输入第二个学生的成绩:");
scanf("%f", &std2.score);
// 输出学生信息
printf("第一个学生的姓名:%s\n", std1.name);
printf("第一个学生的年龄:%d\n", std1.age);
printf("第一个学生的成绩:%.2f\n", std1.score);
printf("第二个学生的姓名:%s\n", std2.name);
printf("第二个学生的年龄:%d\n", std2.age);
printf("第二个学生的成绩:%.2f\n", std2.score);
return 0;
}
这段代码是一个简单的包含结构体的C语言程序。首先定义了一个名为Student的结构体,包含了姓名、年龄和成绩三个成员变量。然后在main函数中声明了两个Student类型的对象std1和std2。接下来,通过scanf函数从用户输入中获取第一个学生的信息,并存储到std1中;再通过scanf函数获取第二个学生的信息,并存储到std2中。最后,通过printf函数将两个学生的信息输出到屏幕上。代码执行完毕后,程序将返回0,表示执行成功。
### 回答3:
下面是一个包含结构体的C语言代码示例:
```c
#include <stdio.h>
// 定义一个结构体表示学生信息
struct Student {
char name[20];
int age;
float score;
};
int main() {
// 创建一个学生对象并初始化
struct Student stu1 = {"小明", 18, 95.5};
// 输出学生信息
printf("学生姓名:%s\n", stu1.name);
printf("学生年龄:%d\n", stu1.age);
printf("学生成绩:%.1f\n", stu1.score);
// 修改学生信息
stu1.age = 19;
stu1.score = 98.0;
// 输出修改后的学生信息
printf("修改后的学生年龄:%d\n", stu1.age);
printf("修改后的学生成绩:%.1f\n", stu1.score);
return 0;
}
```
以上代码定义了一个包含三个成员变量(姓名、年龄、成绩)的结构体`Student`,并在`main`函数中创建一个`stu1`的学生对象,并初始化其成员变量。然后,通过`printf`函数依次输出学生姓名、年龄、成绩。之后,修改学生的年龄和成绩,并通过`printf`函数输出修改后的信息。输出的结果如下:
```
学生姓名:小明
学生年龄:18
学生成绩:95.5
修改后的学生年龄:19
修改后的学生成绩:98.0
```
这样,就完成了一个包含结构体的C语言代码示例。
相关推荐
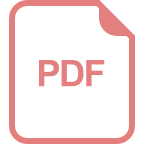
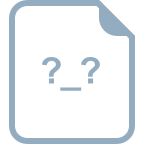
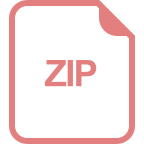














