package czx; import java.util.Arrays; public class hs { static final int N = 4; static final int b = 13; static int []v = {12,11,9,8}; static int []w = {8,6,4,3}; static int cv; static int cw; static int bestValue; static int []x = new int[4]; static int []bestX = new int[4]; static int count = 0; public static void main(String[] args) { BackTrackingKnapsack(0); System.out.println("物品的价值:"+Arrays.toString(v)); System.out.println("物品的重量:"+Arrays.toString(w)); System.out.println("最大价值为: " + bestValue); { System.out.println("输出的Y值为" + Arrays.toString(bestX)); }System.out.println("算法的运行次数为:" + count); } public static void BackTrackingKnapsack(int k) { if (k > N-1) { count ++; if (bestValue < cv) { bestValue = cv;count ++; for (int i = 0; i < N; i++) { bestX[i] = x[i];count ++; } } } else { for (int i = 0; i <= 1; i++) { x[k] = i;count ++; if (i == 0) { BackTrackingKnapsack(k+1);count ++; } else { if (cw + w[k] <= b) {count ++; cw += w[k];count ++; cv += v[k];count ++; BackTrackingKnapsack(k+1); cw -= w[k]; count ++; cv -= v[k];count ++; } } } } } }
时间: 2024-01-27 21:05:13 浏览: 24
这段代码有一些可以优化的地方,以下是一些建议:
1. 变量命名:建议使用有意义的变量名,例如将 `cv` 改为 `currentValue`,将 `cw` 改为 `currentWeight`。
2. 循环次数:在循环中使用了递归,可能导致算法的运行次数很大,可以尝试使用循环代替递归。
3. 函数返回值:函数 `BackTrackingKnapsack` 没有返回值,可以考虑将结果存储在一个全局变量中,或者将它改为返回一个结果。
4. 代码风格:可以考虑使用缩进和空格来使代码更易读。
下面是一个可能的优化版本:
```java
package czx;
import java.util.Arrays;
public class hs {
static final int N = 4;
static final int b = 13;
static int[] v = {12, 11, 9, 8};
static int[] w = {8, 6, 4, 3};
static int currentValue;
static int currentWeight;
static int bestValue;
static int[] x = new int[4];
static int[] bestX = new int[4];
static int count = 0;
public static void main(String[] args) {
backTrackingKnapsack();
System.out.println("物品的价值:" + Arrays.toString(v));
System.out.println("物品的重量:" + Arrays.toString(w));
System.out.println("最大价值为: " + bestValue);
System.out.println("输出的Y值为" + Arrays.toString(bestX));
System.out.println("算法的运行次数为:" + count);
}
public static void backTrackingKnapsack() {
int k = 0;
while (k >= 0) {
if (k >= N) {
if (bestValue < currentValue) {
bestValue = currentValue;
System.arraycopy(x, 0, bestX, 0, N);
}
k--;
} else {
count++;
x[k] = 0;
if (currentWeight + w[k] <= b) {
x[k] = 1;
currentWeight += w[k];
currentValue += v[k];
}
if (currentWeight <= b && getUpperBound(k) > bestValue) {
k++;
} else {
currentWeight -= x[k] * w[k];
currentValue -= x[k] * v[k];
k--;
}
}
}
}
private static double getUpperBound(int k) {
double bound = currentValue;
int weight = currentWeight;
for (int i = k; i < N; i++) {
if (weight + w[i] <= b) {
weight += w[i];
bound += v[i];
} else {
bound += (b - weight) * (v[i] / (double) w[i]);
break;
}
}
return bound;
}
}
```
此版本中,使用了循环代替了递归,将变量名更改为更有意义的名称,删除了一些无用的计数器,添加了一个上界函数,可以提高算法的效率。
相关推荐
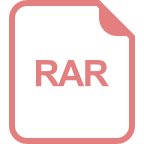












