霍夫变换直线检测程序c语言
时间: 2024-06-16 10:06:44 浏览: 18
霍夫变换直线检测是一种常用的图像处理算法,用检测图像中的直线。下面是一个使用C语言实现的简单霍夫变换直线检测的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define MAX_VOTES 1000
typedef struct {
int x;
int y;
} Point;
typedef struct {
float rho;
float theta;
} Line;
void houghTransform(int width, int height, unsigned char* image, int threshold, Line* lines, int* numLines) {
int maxRho = sqrt(width * width + height * height);
int numThetas = 180;
int* accumulator = (int*)calloc(maxRho * numThetas, sizeof(int));
// Voting
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
if (image[y * width + x] > 0) {
for (int theta = 0; theta < numThetas; theta++) {
float angle = theta * M_PI / numThetas;
float rho = x * cos(angle) + y * sin(angle);
int rhoIndex = (int)(rho + maxRho / 2);
accumulator[rhoIndex * numThetas + theta]++;
}
}
}
}
// Finding lines
*numLines = 0;
for (int rhoIndex = 0; rhoIndex < maxRho; rhoIndex++) {
for (int theta = 0; theta < numThetas; theta++) {
if (accumulator[rhoIndex * numThetas + theta] > threshold) {
lines[*numLines].rho = rhoIndex - maxRho / 2;
lines[*numLines].theta = theta * M_PI / numThetas;
(*numLines)++;
if (*numLines >= MAX_VOTES) {
return;
}
}
}
}
free(accumulator);
}
int main() {
int width = 640;
int height = 480;
unsigned char* image = (unsigned char*)malloc(width * height * sizeof(unsigned char));
// Load image data here
Line lines[MAX_VOTES];
int numLines = 0;
int threshold = 100;
houghTransform(width, height, image, threshold, lines, &numLines);
printf("Detected %d lines:\n", numLines);
for (int i = 0; i < numLines; i++) {
printf("Line %d: rho = %.2f, theta = %.2f\n", i+1, lines[i].rho, lines[i].theta);
}
free(image);
return 0;
}
```
这个程序实现了一个简单的霍夫变换直线检测算法。它首先对输入的图像进行霍夫变换,然后根据设定的阈值找到符合条件的直线,并输出检测到的直线的参数(rho和theta)。
相关推荐
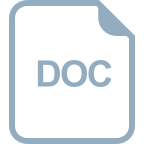
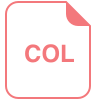













