Java中读取txt文件,并且判断文件的编码是不是UTF-8格式,如果不是UTF-8转换成UTF-8格式
时间: 2024-09-09 09:03:51 浏览: 62
在Java中,我们可以使用`java.nio.charset`包下的工具来检测并转换文件的编码。以下是一个示例,展示了如何读取TXT文件、检查其编码并转成UTF-8:
```java
import java.nio.charset.Charset;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Paths;
public class ReadAndDecodeFile {
public static void main(String[] args) {
try {
// 文件路径
String filePath = "/path/to/your/text.txt";
// 读取文件内容
byte[] bytes = Files.readAllBytes(Paths.get(filePath));
// 判断原始编码
Charset originalCharset = detectOriginalCharset(bytes);
if (!originalCharset.equals(StandardCharsets.UTF_8)) {
// 如果不是UTF-8,尝试转换为UTF-8
byte[] decodedBytes = originalCharset.decode(bytes).encode(StandardCharsets.UTF_8).array();
// 写回新的UTF-8编码的文件
Files.write(Paths.get(filePath), decodedBytes, StandardCharsets.UTF_8);
System.out.println("Original encoding was not UTF-8, converted and saved as UTF-8.");
} else {
System.out.println("File is already in UTF-8 format.");
}
} catch (IOException e) {
System.err.println("Error reading or writing the file: " + e.getMessage());
e.printStackTrace();
}
}
private static Charset detectOriginalCharset(byte[] bytes) {
for(Charset charset : Charset.availableCharsets().values()) {
try {
if (charset.canDecode().test(bytes)) {
return charset; // 返回能解码此字节序列的第一个字符集
}
} catch (CharacterCodingException ex) {
continue;
}
}
throw new IllegalArgumentException("Could not determine original encoding of the file");
}
}
```
这个程序首先读取文件内容为字节数组,然后遍历所有已知的字符集,找到能成功解码原始字节的那一种。如果找到的是非UTF-8编码,就将其转换为UTF-8并保存回去。
阅读全文
相关推荐














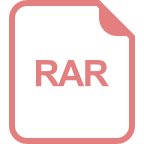



