用Java程序写一个选择排序对班级期末考语数英三科成绩进行排序(要求是创建成绩类)
时间: 2024-09-07 12:05:38 浏览: 52
选择排序是一种简单直观的排序算法,它的工作原理是每次从待排序的数据元素中选出最小(或最大)的一个元素,存放在序列的起始位置,直到全部待排序的数据元素排完。在Java中实现选择排序,首先我们需要定义一个成绩类,然后编写选择排序的逻辑来对学生的成绩进行排序。
下面是一个简单的示例代码,包括成绩类(Score)的定义和使用选择排序算法对成绩进行排序:
```java
// 成绩类,包含学生姓名和三科成绩
class Score {
String name;
int chinese;
int math;
int english;
public Score(String name, int chinese, int math, int english) {
this.name = name;
this.chinese = chinese;
this.math = math;
this.english = english;
}
// 重写toString方法,方便打印
@Override
public String toString() {
return "Score{" +
"name='" + name + '\'' +
", chinese=" + chinese +
", math=" + math +
", english=" + english +
'}';
}
}
// 选择排序算法
public class SelectionSort {
public static void main(String[] args) {
Score[] scores = {
new Score("张三", 80, 90, 70),
new Score("李四", 85, 75, 65),
new Score("王五", 90, 80, 70)
};
// 对成绩进行排序
selectSort(scores);
// 输出排序后的结果
for (Score score : scores) {
System.out.println(score);
}
}
// 选择排序方法
public static void selectSort(Score[] arr) {
for (int i = 0; i < arr.length - 1; i++) {
// 假设当前位置为最小值的索引
int minIndex = i;
for (int j = i + 1; j < arr.length; j++) {
// 比较当前索引位置的值与后面的值,找到最小值的索引
if (arr[j].chinese < arr[minIndex].chinese) { // 这里假设按语文成绩排序
minIndex = j;
}
}
// 交换最小值的索引和当前位置的索引
if (i != minIndex) {
Score temp = arr[i];
arr[i] = arr[minIndex];
arr[minIndex] = temp;
}
}
}
}
```
在这个示例中,我们定义了一个`Score`类,它包含了学生的姓名和三科成绩。在`main`方法中,我们创建了一个`Score`数组来存储三个学生的成绩,并使用`selectSort`方法对成绩进行排序。排序是基于学生的语文成绩进行的,如果需要按照其他科目的成绩进行排序,只需更改`selectSort`方法中比较的字段即可。
相关推荐
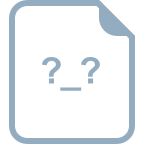
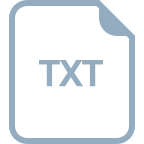
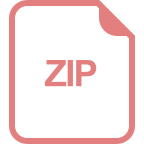














