使用c语言,编写一个内核模块,内核创建100个内核线程,并在结束之后停止100个内核线程,
时间: 2024-10-15 20:16:34 浏览: 3
在Linux内核编程中,创建和管理内核线程(kthread)通常需要使用`kernel_thread()`函数,这是一个从`linux/kernel.h`头文件导出的宏,用于快速创建一个新的内核任务。以下是简单的步骤概述:
首先,你需要包含必要的头文件并初始化内核模块:
```c
#include <linux/module.h>
#include <linux/kernel.h>
#include <linux/kthread.h>
#include <linux/sched.h>
```
接下来,你可以定义一个内核线程函数,它将在新的线程中运行:
```c
static int my_thread_fn(void *data)
{
// 在这里放置你的线程执行的代码
printk(KERN_INFO "Thread %d started.\n", data);
// ... 线程业务逻辑
printk(KERN_INFO "Thread %d finished.\n", data);
return 0;
}
```
然后,创建100个线程的函数可以像下面这样:
```c
static void create_threads(void)
{
for (int i = 0; i < 100; i++) {
struct task_struct *tid = kthread_create(my_thread_fn, &i, "my_thread-%d");
if (IS_ERR(tid)) {
printk(KERN_ERR "Failed to create thread %d\n", i);
continue;
}
wake_up_process(tid);
}
}
```
最后,在模块的`sys_exit()`或其他适当的退出点上,停止所有线程:
```c
static void stop_threads(void)
{
for (int i = 0; i < 100; i++) {
struct task_struct *tid = find_task_by_pid(i); // 假设线程PID为i
if (!tid) {
printk(KERN_ERR "Failed to find thread %d\n", i);
continue;
}
wake_up_process(tid);
wait_for_completion(&tid->wait);
put_task_struct(tid);
}
}
```
记得要在`module_init()`和`module_exit()`函数中注册`create_threads`和`stop_threads`。
相关推荐
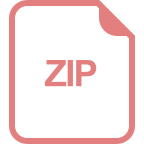
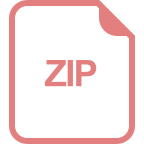
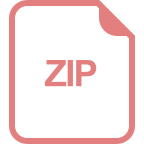







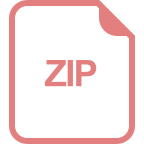
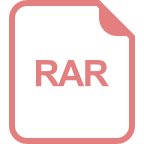
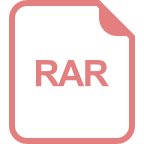
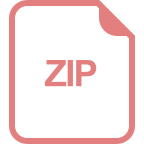
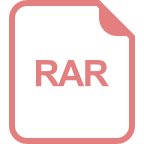
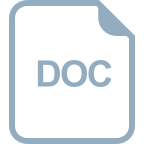
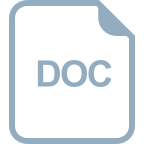
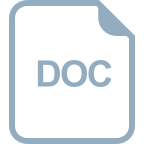
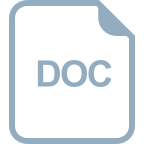