用vhdl语言生成一个4*4矩阵键盘扫描
时间: 2023-07-24 22:11:39 浏览: 46
下面是一个简单的 VHDL 代码实现,用于生成一个 4x4 的矩阵键盘扫描器:
```vhdl
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
use IEEE.STD_LOGIC_UNSIGNED.ALL;
entity keypad is
Port (
row : inout std_logic_vector(3 downto 0); -- 行信号
col : in std_logic_vector(3 downto 0); -- 列信号
key : out std_logic_vector(15 downto 0) -- 按键状态
);
end keypad;
architecture Behavioral of keypad is
signal row_int : std_logic_vector(3 downto 0); -- 内部行信号
signal col_int : std_logic_vector(3 downto 0); -- 内部列信号
begin
-- 行信号输出
row <= row_int;
-- 列信号输入
col_int <= col;
-- 按键状态输出
key(0) <= not(row_int(0)) and not(col_int(0));
key(1) <= not(row_int(0)) and not(col_int(1));
key(2) <= not(row_int(0)) and not(col_int(2));
key(3) <= not(row_int(0)) and not(col_int(3));
key(4) <= not(row_int(1)) and not(col_int(0));
key(5) <= not(row_int(1)) and not(col_int(1));
key(6) <= not(row_int(1)) and not(col_int(2));
key(7) <= not(row_int(1)) and not(col_int(3));
key(8) <= not(row_int(2)) and not(col_int(0));
key(9) <= not(row_int(2)) and not(col_int(1));
key(10) <= not(row_int(2)) and not(col_int(2));
key(11) <= not(row_int(2)) and not(col_int(3));
key(12) <= not(row_int(3)) and not(col_int(0));
key(13) <= not(row_int(3)) and not(col_int(1));
key(14) <= not(row_int(3)) and not(col_int(2));
key(15) <= not(row_int(3)) and not(col_int(3));
-- 行扫描控制
process(col_int)
begin
row_int <= "1110"; -- 初始化为第一行
wait for 10 ns; -- 等待一段时间
row_int <= "1101"; -- 扫描第二行
wait for 10 ns; -- 等待一段时间
row_int <= "1011"; -- 扫描第三行
wait for 10 ns; -- 等待一段时间
row_int <= "0111"; -- 扫描第四行
wait for 10 ns; -- 等待一段时间
end process;
end Behavioral;
```
该代码使用了一个 `process` 进程来控制行信号的扫描,通过改变 `row_int` 的值来控制行信号的输出,从而实现对矩阵键盘的扫描。同时,还使用了 `wait for` 语句来控制每次扫描的持续时间。最后,通过对 `row_int` 和 `col_int` 的取反运算,实现对按键状态的检测和输出。
相关推荐
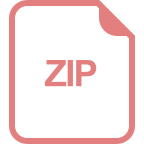
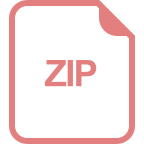
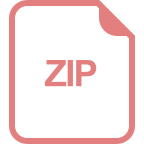














