使用终端A上的扩展IO口PA3连接DS18B20温度传感器。 每当按键ASW4按下,通过串口上报温度数据一次,温度发送格式: "C:25.26\r\n" 串口发送格式(字符串) 备注: C为大写字母,温度值结果保留小数点后2位有效数字。 串口发送的字符串应包含换行、回车符号:"\r\n"。 通过USART3的USB转串口功能实现,波特率设置为9600 bps。
时间: 2024-03-17 20:39:37 浏览: 55
这是一个需要编写嵌入式程序的问题,需要使用编程语言和相应的开发环境来完成。以下是一个基于STM32F4开发板的C语言代码示例,实现了该功能:
```c
#include "stm32f4xx.h"
#include <stdio.h>
#include <string.h>
#define DS18B20_PIN GPIO_Pin_3
#define DS18B20_GPIO GPIOA
void delay_us(uint32_t us)
{
for (uint32_t i = 0; i < us * 8; i++);
}
void ds18b20_init(void)
{
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = DS18B20_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_OType = GPIO_OType_OD;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(DS18B20_GPIO, &GPIO_InitStructure);
GPIO_SetBits(DS18B20_GPIO, DS18B20_PIN);
}
void ds18b20_write_bit(uint8_t bit)
{
GPIO_ResetBits(DS18B20_GPIO, DS18B20_PIN);
delay_us(2);
if (bit)
{
GPIO_SetBits(DS18B20_GPIO, DS18B20_PIN);
delay_us(60);
}
else
{
delay_us(60);
GPIO_SetBits(DS18B20_GPIO, DS18B20_PIN);
}
delay_us(2);
}
void ds18b20_write_byte(uint8_t byte)
{
for (uint8_t i = 0; i < 8; i++)
{
ds18b20_write_bit(byte & 0x01);
byte >>= 1;
}
}
uint8_t ds18b20_read_bit(void)
{
GPIO_ResetBits(DS18B20_GPIO, DS18B20_PIN);
delay_us(2);
GPIO_SetBits(DS18B20_GPIO, DS18B20_PIN);
delay_us(12);
GPIO_PinState state = GPIO_ReadInputDataBit(DS18B20_GPIO, DS18B20_PIN);
delay_us(50);
return state;
}
uint8_t ds18b20_read_byte(void)
{
uint8_t byte = 0;
for (uint8_t i = 0; i < 8; i++)
{
byte |= ds18b20_read_bit() << i;
}
return byte;
}
float ds18b20_read_temperature(void)
{
ds18b20_init();
ds18b20_write_byte(0xCC);
ds18b20_write_byte(0x44);
while (!ds18b20_read_bit());
ds18b20_init();
ds18b20_write_byte(0xCC);
ds18b20_write_byte(0xBE);
uint16_t raw = ds18b20_read_byte() | (ds18b20_read_byte() << 8);
int16_t temp = (int16_t)raw / 16;
float dec = (float)(raw & 0x0F) / 16;
float temperature = (float)temp + dec;
return temperature;
}
void usart3_init(uint32_t baudrate)
{
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART3, ENABLE);
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOB, ENABLE);
GPIO_PinAFConfig(GPIOB, GPIO_PinSource10, GPIO_AF_USART3);
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOB, &GPIO_InitStructure);
USART_InitTypeDef USART_InitStructure;
USART_InitStructure.USART_BaudRate = baudrate;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Tx;
USART_Init(USART3, &USART_InitStructure);
USART_Cmd(USART3, ENABLE);
}
void usart3_send_string(char* str)
{
while (*str)
{
USART_SendData(USART3, *str++);
while (USART_GetFlagStatus(USART3, USART_FLAG_TXE) == RESET);
}
}
int main(void)
{
usart3_init(9600);
while (1)
{
if (GPIO_ReadInputDataBit(GPIOC, GPIO_Pin_1) == RESET)
{
float temperature = ds18b20_read_temperature();
char str[16];
sprintf(str, "C:%.2f\r\n", temperature);
usart3_send_string(str);
while (GPIO_ReadInputDataBit(GPIOC, GPIO_Pin_1) == RESET);
}
}
}
```
该程序首先初始化了DS18B20温度传感器和USART3串口。在主循环中,程序不断读取ASW4按键状态,如果按键被按下,则读取温度传感器的温度值,并通过USART3串口发送给上位机。发送的字符串格式为"C:25.26\r\n",其中温度值使用sprintf函数转换为字符串格式。发送完成后,程序等待ASW4按键释放。
阅读全文
相关推荐
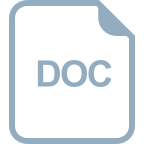
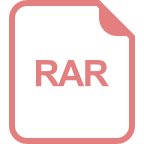
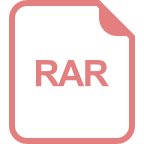
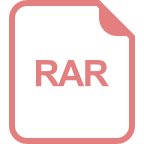
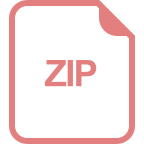
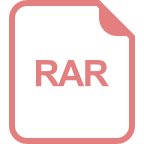
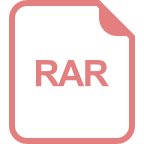
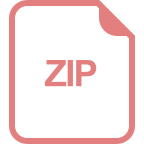
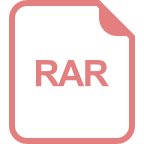
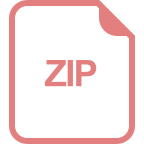
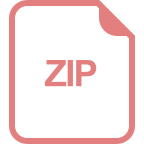
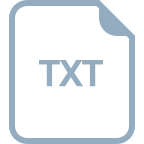
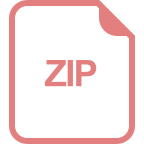
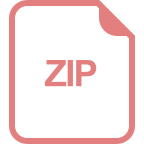
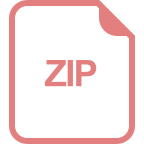
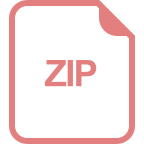