java定义一个二维数组,从键盘输入两个矩阵,求两个矩阵的加法、减法、乘积,并输出到屏幕
时间: 2024-10-13 14:10:39 浏览: 68
在Java中,你可以通过定义一个二维数组来存储矩阵数据,然后让用户从键盘输入两个矩阵的元素。对于矩阵运算,如加法、减法和乘法,可以创建相应的函数来处理。下面是一个简单的示例:
```java
import java.util.Scanner;
public class MatrixOperations {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// 定义矩阵大小
int rows, cols;
System.out.print("请输入矩阵行数: ");
rows = scanner.nextInt();
System.out.print("请输入矩阵列数: ");
cols = scanner.nextInt();
// 创建矩阵数组
double[][] matrixA = new double[rows][cols];
double[][] matrixB = new double[rows][cols];
// 输入矩阵A
System.out.println("请输入矩阵A:");
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
matrixA[i][j] = scanner.nextDouble();
}
}
// 输入矩阵B
System.out.println("请输入矩阵B:");
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
matrixB[i][j] = scanner.nextDouble();
}
}
// 矩阵操作
double[][] sum = addMatrices(matrixA, matrixB);
double[][] difference = subtractMatrices(matrixA, matrixB);
double[][] product = multiplyMatrices(matrixA, matrixB);
// 输出结果
System.out.println("\n矩阵A + B:");
printMatrix(sum);
System.out.println("\n矩阵A - B:");
printMatrix(difference);
System.out.println("\n矩阵A * B:");
printMatrix(product);
}
// 加法函数
public static double[][] addMatrices(double[][] a, double[][] b) {
return new double[a.length][a[0].length] {
@Override
public double get(int row, int col) {
return a[row][col] + b[row][col];
}
};
}
// 减法函数
public static double[][] subtractMatrices(double[][] a, double[][] b) {
return new double[a.length][a[0].length] {
@Override
public double get(int row, int col) {
return a[row][col] - b[row][col];
}
};
}
// 乘法函数,这里假设两个矩阵相乘后的结果也是同维度
public static double[][] multiplyMatrices(double[][] a, double[][] b) {
double[][] result = new double[a.length][b[0].length];
for (int i = 0; i < a.length; i++) {
for (int j = 0; j < b[0].length; j++) {
for (int k = 0; k < a[0].length; k++) {
result[i][j] += a[i][k] * b[k][j];
}
}
}
return result;
}
// 打印矩阵
private static void printMatrix(double[][] matrix) {
for (double[] row : matrix) {
for (double value : row) {
System.out.print(value + " ");
}
System.out.println();
}
}
}
```
阅读全文
相关推荐
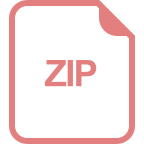
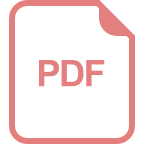
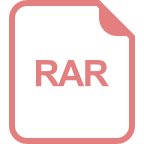


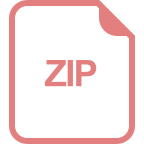
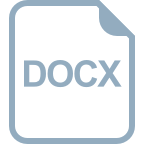
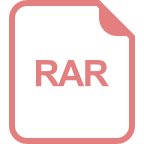
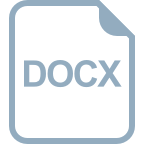
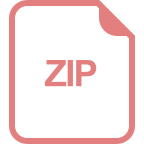
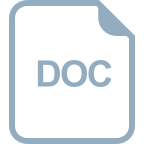
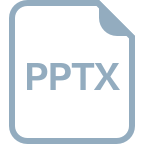
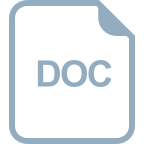
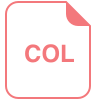
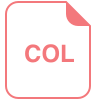



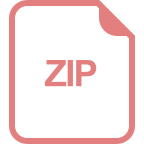