用python实现手写数字识别
时间: 2023-09-18 10:13:17 浏览: 43
实现手写数字识别可以使用深度学习框架,比如TensorFlow或PyTorch。以下是一个基于TensorFlow的手写数字识别示例代码:
1. 准备数据集
首先需要准备手写数字的图片数据集,可以使用MNIST数据集,也可以自己制作。这里以MNIST为例,使用TensorFlow内置的数据集加载器来加载数据集。
```python
import tensorflow as tf
# 加载MNIST数据集
mnist = tf.keras.datasets.mnist
(train_images, train_labels), (test_images, test_labels) = mnist.load_data()
```
2. 数据预处理
将图片数据转换为模型需要的格式,并将像素值归一化到[0, 1]范围内。
```python
# 将像素值归一化到[0, 1]范围内
train_images, test_images = train_images / 255.0, test_images / 255.0
# 将图片数据转换为模型需要的格式
train_images = train_images.reshape(-1, 28, 28, 1)
test_images = test_images.reshape(-1, 28, 28, 1)
```
3. 定义模型
使用卷积神经网络(CNN)来构建模型,可以使用TensorFlow的Sequential模型来定义模型。
```python
from tensorflow.keras import layers
# 定义模型
model = tf.keras.Sequential([
layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation='relu'),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation='relu'),
layers.Flatten(),
layers.Dense(64, activation='relu'),
layers.Dense(10)
])
```
4. 编译模型
使用TensorFlow的compile方法来编译模型,指定损失函数、优化器和评价指标。
```python
model.compile(optimizer='adam',
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
```
5. 训练模型
使用TensorFlow的fit方法来训练模型,指定训练数据、标签、批次大小和训练轮数。
```python
model.fit(train_images, train_labels, epochs=5, batch_size=64)
```
6. 评估模型
使用TensorFlow的evaluate方法来评估模型性能,指定测试数据和标签。
```python
test_loss, test_acc = model.evaluate(test_images, test_labels, verbose=2)
print("Test accuracy:", test_acc)
```
7. 使用模型进行预测
使用TensorFlow的predict方法来使用训练好的模型进行预测,指定测试数据。
```python
import numpy as np
# 随机选择一张测试图片进行预测
index = np.random.randint(len(test_images))
image = test_images[index]
label = test_labels[index]
print("Label:", label)
# 对测试图片进行预测
predictions = model.predict(np.array([image]))
predicted_label = np.argmax(predictions[0])
print("Predicted label:", predicted_label)
```
相关推荐
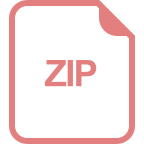
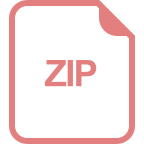












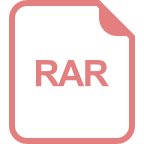