本题目构造一棵二叉检索树。要求读入n个整数,以0结束。最后输出这棵树的先序序列。用C语言
时间: 2023-12-21 08:04:35 浏览: 69
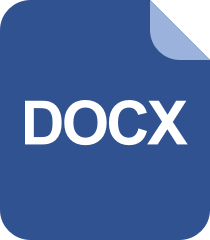
用C语言编写二叉排序树
以下一种可能的解法,使用递归构造二叉搜索树,然后输出先序遍历序列:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树节点结构体
typedef struct TreeNode {
int val;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
// 插入节点
TreeNode* insert(TreeNode* root, int val) {
if (root == NULL) {
TreeNode* node = (TreeNode*)malloc(sizeof(TreeNode));
node->val = val;
node->left = NULL;
node->right = NULL;
return node;
}
if (val < root->val) {
root->left = insert(root->left, val);
} else {
root->right = insert(root->right, val);
}
return root;
}
// 先序遍历
void preorder(TreeNode* root) {
if (root == NULL) {
return;
}
printf("%d ", root->val);
preorder(root->left);
preorder(root->right);
}
int main() {
TreeNode* root = NULL;
int val;
while (1) {
scanf("%d", &val);
if (val == 0) {
break;
}
root = insert(root, val);
}
preorder(root);
return 0;
}
```
使用示例:
输入:
```
5 3 1 4 7 6 8 0
```
输出:
```
5 3 1 4 7 6 8
```
阅读全文
相关推荐
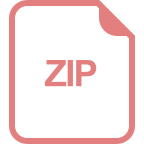















