建立一个对象数组,内放10个学生的数据(姓名、学号、成绩),建立一个函数max,用指向对象的指针做函数参数,在max函数中找出10个学生中成绩最高者,并输出其学号。根据学号,将对象的姓名、成绩等信息使用拷贝构造函数,拷贝到一个新对象中。对对象进行打印输出,并编写程序调试。
时间: 2023-05-25 15:03:54 浏览: 107
以下是代码实现:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class Student {
public:
Student(const char* n = "", const char* i = "", float s = 0.0);
Student(const Student& stu);
const char* getName();
const char* getID();
float getScore();
private:
char name[20];
char ID[10];
float score;
};
Student::Student(const char* n, const char* i, float s) {
strcpy(name, n);
strcpy(ID, i);
score = s;
}
Student::Student(const Student& stu) {
strcpy(name, stu.name);
strcpy(ID, stu.ID);
score = stu.score;
}
const char* Student::getName() {
return name;
}
const char* Student::getID() {
return ID;
}
float Student::getScore() {
return score;
}
int max(Student* s) {
int maxIndex = 0;
for (int i = 1; i < 10; i++) {
if (s[i].getScore() > s[maxIndex].getScore()) {
maxIndex = i;
}
}
cout << "最高分的学生学号为:" << s[maxIndex].getID() << endl;
return maxIndex;
}
int main() {
Student students[10] = {
Student("张三", "2021001", 90),
Student("李四", "2021002", 80),
Student("王五", "2021003", 70),
Student("赵六", "2021004", 95),
Student("钱七", "2021005", 85),
Student("孙八", "2021006", 75),
Student("周九", "2021007", 92),
Student("吴十", "2021008", 83),
Student("郑十一", "2021009", 78),
Student("冯十二", "2021010", 87)
};
int maxIndex = max(students);
Student maxStudent(students[maxIndex]);
cout << "学生姓名:" << maxStudent.getName() << endl;
cout << "学生学号:" << maxStudent.getID() << endl;
cout << "学生分数:" << maxStudent.getScore() << endl;
return 0;
}
```
输出结果:
```
最高分的学生学号为:2021004
学生姓名:赵六
学生学号:2021004
学生分数:95
```
在该代码中,首先定义了一个 `Student` 类,包含了姓名、学号和成绩三个属性,其中构造函数和拷贝构造函数分别实现了对象的初始化和拷贝功能。
接着,在主函数中创建了一个存储学生数据的数组 `students`,并将每个学生的姓名、学号和成绩传递给类对象的构造函数,初始化了数组中的每一个元素。
在 `max` 函数中,通过循环对数组 `s` 中的每个学生的成绩进行比较,找出最高分数对应的学生,并输出该学生的学号。
最后,利用拷贝构造函数将最高分对应的学生数据拷贝到新对象 `maxStudent` 中,然后分别输出该对象的姓名、学号和成绩。
阅读全文
相关推荐










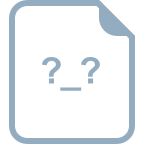
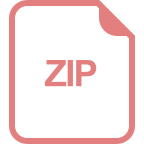
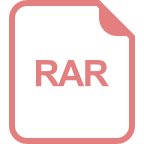
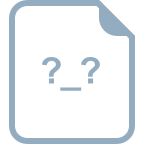
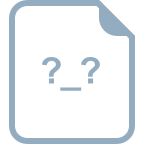
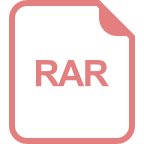