可以向我展示一段包含unix 高级编程知识点的代码,要求使用open()、close()、read()、write()、lseek(),并在代码中使用注释详细解释这些函数的每一个参数
时间: 2023-03-01 19:29:13 浏览: 59
好的,以下是一段使用 unix 高级编程中的 open()、close()、read()、write()、lseek() 的代码。
```
#include <fcntl.h>
#include <unistd.h>
int main() {
// 使用 open() 函数打开文件,并返回文件描述符
int fd = open("example.txt", O_RDWR | O_CREAT, 0666);
if (fd == -1) {
perror("open error");
return 1;
}
// 使用 write() 函数向文件中写入数据
const char* message = "Hello, World!\n";
ssize_t written_bytes = write(fd, message, strlen(message));
if (written_bytes == -1) {
perror("write error");
close(fd);
return 1;
}
// 使用 lseek() 函数移动文件指针到文件开头
off_t offset = lseek(fd, 0, SEEK_SET);
if (offset == -1) {
perror("lseek error");
close(fd);
return 1;
}
// 使用 read() 函数从文件中读取数据
char buffer[1024];
ssize_t read_bytes = read(fd, buffer, sizeof(buffer));
if (read_bytes == -1) {
perror("read error");
close(fd);
return 1;
}
// 使用 close() 函数关闭文件
int result = close(fd);
if (result == -1) {
perror("close error");
return 1;
}
// 输出读取到的数据
printf("Read from file: %s", buffer);
return 0;
}
```
以下是参数的详细解释:
- open() 函数:
- 第一个参数是文件名称。
- 第二个参数是打开模式,可以使用 O_RDONLY、O_WRONLY、O_RDWR 之一,也可以使用 | 运算符与其他标志位结合,例如 O_RDWR | O_CREAT 表示以读写模式打开,如果文件不
相关推荐





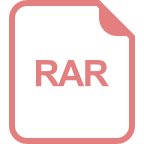
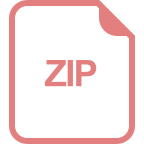
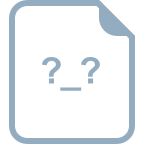
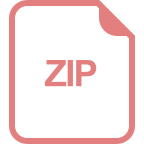
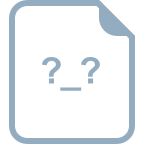
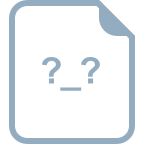