vue3,光标所在位置插入字符,没有选中光标的话默认在输入框最前面添加字符
时间: 2023-08-31 09:41:17 浏览: 80
### 回答1:
vue3 中要在光标所在位置插入字符,可以使用 $refs 和 selectionEnd 属性。
首先,你需要在组件的 template 中给输入框加上一个 ref 属性,例如:
```html
<template>
<input ref="input" />
</template>
```
然后,你就可以在组件中通过 this.$refs.input 访问到输入框的 DOM 元素了。你可以使用 selectionEnd 属性获取光标的位置。
假设你想在光标所在位置插入字符 "x",可以这样做:
```javascript
insertChar(char) {
// 获取输入框的 DOM 元素
const input = this.$refs.input;
// 获取光标的位置
const pos = input.selectionEnd;
// 在光标处插入字符
input.value = input.value.slice(0, pos) + char + input.value.slice(pos);
// 设置光标的位置
input.selectionEnd = pos + 1;
}
```
如果没有选中的光标的话,就默认在输入框最前面添加字符,可以这样做:
```javascript
insertChar(char) {
// 获取输入框的 DOM 元素
const input = this.$refs.input;
// 获取光标的位置,如果没有选中的光标就将光标位置设为 0
const pos = input.selectionEnd || 0;
// 在光标处插入字符
input.value = input.value.slice(0, pos) + char + input.value.slice(pos);
// 设置光标的位置
input.selectionEnd = pos + 1;
}
```
希望这能帮到你!
### 回答2:
在Vue3中,要在光标位置插入字符,可以通过以下步骤实现。首先,获取当前输入框的值和光标位置,可以使用`event.target.value`和`event.target.selectionStart`属性来获取值和光标位置。
接下来,判断光标是否有选中文本。如果有选中文本,可以使用`substring`函数将选中文本前面和后面的内容拼接起来,然后再在中间插入需要添加的字符。
如果光标没有选中文本,说明需要在输入框最前面添加字符。这时可以直接在当前值的前面拼接需要添加的字符。
最后,更新输入框的值,并且将光标位置设置为新插入字符的后面。可以使用`event.target.value`来更新输入框的值,然后使用`event.target.setSelectionRange()`函数将光标位置设置为新插入字符的后面。
以上步骤可以用以下代码实现:
```javascript
insertCharacter(event, character) {
const input = event.target;
const currentValue = input.value;
const selectionStart = input.selectionStart;
const selectionEnd = input.selectionEnd;
if (selectionStart !== selectionEnd) {
// 有选中文本
const newContent = currentValue.substring(0, selectionStart) + character + currentValue.substring(selectionEnd);
input.value = newContent;
input.setSelectionRange(selectionStart + 1, selectionStart + 1);
} else {
// 没有选中文本,默认在最前面添加字符
const newContent = character + currentValue;
input.value = newContent;
input.setSelectionRange(1, 1);
}
}
```
这样,无论光标是否选中文本,都可以在光标位置插入字符,并且如果没有选中文本则默认添加在输入框最前面。
### 回答3:
在Vue3中,插入字符到光标所在位置可以通过一些常用的方法来实现。首先,我们可以监听用户进行输入操作时的事件,例如使用`@input`监听输入框的输入事件。然后,在事件处理函数中,我们可以通过获取输入框的`value`属性值,以及光标的当前位置来进行相应的处理。
当光标被选中时,我们可以使用JavaScript中的`substring()`方法来将输入框已有的内容拆分为两部分,然后将插入的字符和两部分内容进行拼接。具体的代码可以如下所示:
```html
<template>
<div>
<input type="text" v-model="inputValue" @input="handleInput" />
</div>
</template>
<script>
export default {
data() {
return {
inputValue: ""
};
},
methods: {
handleInput() {
// 获取输入框的value值
const value = this.inputValue;
// 获取光标的起始位置
const start = event.target.selectionStart;
// 获取光标的结束位置
const end = event.target.selectionEnd;
// 判断光标是否被选中
if (start !== end) {
// 光标被选中时插入字符
const newValue = value.substring(0, start) + "插入的字符" + value.substring(end);
this.inputValue = newValue;
} else {
// 光标未被选中时在输入框最前面添加字符
this.inputValue = "插入的字符" + value;
}
}
}
};
</script>
```
通过上述代码,我们可以实现在Vue3中根据光标的选中状态来插入字符。如果光标未被选中,字符将被添加到输入框的最前面;如果光标被选中,字符将被插入到光标所在位置。
相关推荐
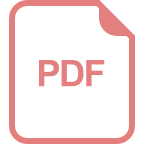








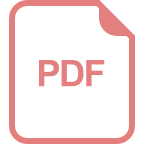
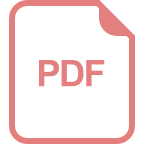
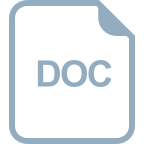
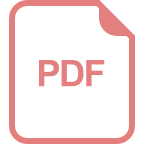
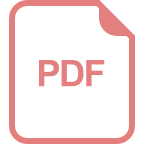
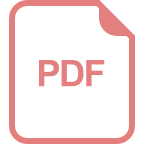