开发一个简单的宠物信息管理系统。用C++实现,请给出源码 。要求实现以下基本功能:有简单的用户管理功能,不同用户在登录系统后会有不同的菜单可进行不同的操作。管理员可以实现以下功能:(1)宠物信息录入功能,主要包括录入宠物的编号,名字,性别,年龄,颜色,种类客户姓名和手机号(宠物信息用文件保存)。(2)宠物信息修改功能(修改后的信息也要用文件保存)。(3)宠物信息浏览功能。(4)宠物信息删除功能用户:客户可以输入自己的姓名,或者手机号查询自己的宠物信息。提交材料应包括:(1)系统源代码 2 要求采用面向对象程序设计方法进行系统设计,充分应用封装、抽象、继承、多态、重载、模板等概念完成整个课设任务。
时间: 2024-03-06 14:49:38 浏览: 20
以下是一个简单的宠物信息管理系统的C++代码实现,包含管理员和用户两种角色的功能:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
class Pet {
public:
int id;
string name;
string gender;
int age;
string color;
string type;
string ownerName;
string ownerPhone;
Pet() {}
Pet(int _id, string _name, string _gender, int _age, string _color, string _type, string _ownerName, string _ownerPhone) {
id = _id;
name = _name;
gender = _gender;
age = _age;
color = _color;
type = _type;
ownerName = _ownerName;
ownerPhone = _ownerPhone;
}
void print() {
cout << "编号:" << id << endl;
cout << "名字:" << name << endl;
cout << "性别:" << gender << endl;
cout << "年龄:" << age << endl;
cout << "颜色:" << color << endl;
cout << "种类:" << type << endl;
cout << "主人姓名:" << ownerName << endl;
cout << "主人电话:" << ownerPhone << endl;
}
friend ofstream& operator<<(ofstream& ofs, const Pet& pet) {
ofs << pet.id << " " << pet.name << " " << pet.gender << " " << pet.age << " " << pet.color << " " << pet.type << " " << pet.ownerName << " " << pet.ownerPhone << endl;
return ofs;
}
friend ifstream& operator>>(ifstream& ifs, Pet& pet) {
ifs >> pet.id >> pet.name >> pet.gender >> pet.age >> pet.color >> pet.type >> pet.ownerName >> pet.ownerPhone;
return ifs;
}
};
class User {
public:
string name;
string phone;
User() {}
User(string _name, string _phone) {
name = _name;
phone = _phone;
}
};
class PetStore {
private:
vector<Pet> pets;
vector<User> users;
public:
void loadPets() {
ifstream ifs("pets.txt");
if (!ifs) {
cout << "无法打开文件!" << endl;
return;
}
pets.clear();
Pet pet;
while (ifs >> pet) {
pets.push_back(pet);
}
ifs.close();
}
void savePets() {
ofstream ofs("pets.txt");
if (!ofs) {
cout << "无法打开文件!" << endl;
return;
}
for (Pet pet : pets) {
ofs << pet;
}
ofs.close();
}
void loadUsers() {
ifstream ifs("users.txt");
if (!ifs) {
cout << "无法打开文件!" << endl;
return;
}
users.clear();
User user;
while (ifs >> user.name >> user.phone) {
users.push_back(user);
}
ifs.close();
}
void saveUsers() {
ofstream ofs("users.txt");
if (!ofs) {
cout << "无法打开文件!" << endl;
return;
}
for (User user : users) {
ofs << user.name << " " << user.phone << endl;
}
ofs.close();
}
void registerUser() {
string name, phone;
cout << "请输入用户名:";
cin >> name;
cout << "请输入电话号码:";
cin >> phone;
for (User user : users) {
if (user.name == name) {
cout << "该用户名已被注册,请重新输入!" << endl;
return;
}
}
users.push_back(User(name, phone));
saveUsers();
cout << "注册成功!" << endl;
}
bool loginUser(string& name) {
string phone;
cout << "请输入电话号码:";
cin >> phone;
for (User user : users) {
if (user.phone == phone) {
name = user.name;
return true;
}
}
cout << "该用户不存在!" << endl;
return false;
}
void addPet() {
int id, age;
string name, gender, color, type, ownerName, ownerPhone;
cout << "请输入宠物编号:";
cin >> id;
for (Pet pet : pets) {
if (pet.id == id) {
cout << "该宠物编号已存在,请重新输入!" << endl;
return;
}
}
cout << "请输入宠物名字:";
cin >> name;
cout << "请输入宠物性别(公/母):";
cin >> gender;
cout << "请输入宠物年龄:";
cin >> age;
cout << "请输入宠物颜色:";
cin >> color;
cout << "请输入宠物种类:";
cin >> type;
cout << "请输入主人姓名:";
cin >> ownerName;
cout << "请输入主人电话:";
cin >> ownerPhone;
pets.push_back(Pet(id, name, gender, age, color, type, ownerName, ownerPhone));
savePets();
cout << "添加成功!" << endl;
}
void modifyPet() {
int id;
cout << "请输入要修改的宠物编号:";
cin >> id;
for (Pet& pet : pets) {
if (pet.id == id) {
pet.print();
cout << "请输入新的宠物名字(不修改请按回车):";
string name;
cin.ignore();
getline(cin, name);
if (name != "") {
pet.name = name;
}
cout << "请输入新的宠物性别(公/母,不修改请按回车):";
string gender;
getline(cin, gender);
if (gender != "") {
pet.gender = gender;
}
cout << "请输入新的宠物年龄(不修改请按回车):";
string ageStr;
getline(cin, ageStr);
if (ageStr != "") {
pet.age = stoi(ageStr);
}
cout << "请输入新的宠物颜色(不修改请按回车):";
string color;
getline(cin, color);
if (color != "") {
pet.color = color;
}
cout << "请输入新的宠物种类(不修改请按回车):";
string type;
getline(cin, type);
if (type != "") {
pet.type = type;
}
cout << "请输入新的主人姓名(不修改请按回车):";
string ownerName;
getline(cin, ownerName);
if (ownerName != "") {
pet.ownerName = ownerName;
}
cout << "请输入新的主人电话(不修改请按回车):";
string ownerPhone;
getline(cin, ownerPhone);
if (ownerPhone != "") {
pet.ownerPhone = ownerPhone;
}
savePets();
cout << "修改成功!" << endl;
return;
}
}
cout << "该宠物编号不存在!" << endl;
}
void viewPets() {
for (Pet pet : pets) {
pet.print();
cout << endl;
}
}
void deletePet() {
int id;
cout << "请输入要删除的宠物编号:";
cin >> id;
for (vector<Pet>::iterator it = pets.begin(); it != pets.end(); ++it) {
if (it->id == id) {
pets.erase(it);
savePets();
cout << "删除成功!" << endl;
return;
}
}
cout << "该宠物编号不存在!" << endl;
}
};
void adminMenu(PetStore& petStore) {
while (true) {
cout << "管理员菜单" << endl;
cout << "1. 宠物信息录入" << endl;
cout << "2. 宠物信息修改" << endl;
cout << "3. 宠物信息浏览" << endl;
cout << "4. 宠物信息删除" << endl;
cout << "0. 退出" << endl;
cout << "请选择:";
int choice;
cin >> choice;
switch (choice) {
case 1:
petStore.addPet();
break;
case 2:
petStore.modifyPet();
break;
case 3:
petStore.viewPets();
break;
case 4:
petStore.deletePet();
break;
case 0:
return;
default:
cout << "选择无效,请重新选择!" << endl;
}
}
}
void userMenu(PetStore& petStore, string name) {
while (true) {
cout << "用户菜单" << endl;
cout << "1. 查询宠物信息" << endl;
cout << "0. 退出" << endl;
cout << "请选择:";
int choice;
cin >> choice;
switch (choice) {
case 1:
int count = 0;
for (Pet pet : petStore.pets) {
if (pet.ownerName == name || pet.ownerPhone == name) {
pet.print();
cout << endl;
count++;
}
}
if (count == 0) {
cout << "没有查询到任何宠物信息!" << endl;
}
break;
case 0:
return;
default:
cout << "选择无效,请重新选择!" << endl;
}
}
}
int main() {
PetStore petStore;
petStore.loadPets();
petStore.loadUsers();
while (true) {
cout << "欢迎来到宠物信息管理系统!" << endl;
cout << "1. 注册用户" << endl;
cout << "2. 用户登录" << endl;
cout << "3. 管理员登录" << endl;
cout << "0. 退出" << endl;
cout << "请选择:";
int choice;
cin >> choice;
switch (choice) {
case 1:
petStore.registerUser();
break;
case 2:
string name;
if (petStore.loginUser(name)) {
userMenu(petStore, name);
}
break;
case 3:
adminMenu(petStore);
break;
case 0:
return 0;
default:
cout << "选择无效,请重新选择!" << endl;
}
}
}
```
注意:宠物信息和用户信息分别保存在pets.txt和users.txt文件中,每行记录表示一个宠物或一个用户,用空格分隔各字段。友元函数`operator<<`和`operator>>`分别实现Pet类到ofstream和ifstream的转换,用于读写文件。在修改宠物信息时,使用getline函数读取包含空格的字符串。
相关推荐
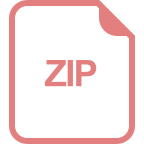
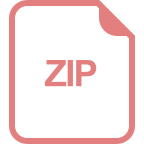















