用c++开发一个简单的宠物信息管理系统,并给出源代码。要求实现以下基本功能:有简单的用户管理功能,不同用户在登录系统后会有不同的菜单可进行不同的操作。管理员可以实现以下功能:(1)宠物信息录入功能,主要包括录入宠物的编号,名字,性别,年龄,颜主要内容色,客户姓名和手机号(宠物信息用文件保存)。(2)宠物信息修改功能(修改后的信息也要用文件保存)。(3)宠物信息浏览功能。(4)宠物信息删除功能 。(5)模糊查询功能。(6)按编号大小排序功能 用户:可以通过姓名,密码登录,进入客户端,客户可以输入自己的姓名,或者手机号查询自己的宠物信息。要求充分应用封装、抽象、继承、多态、重载、模板完成。
时间: 2024-03-19 07:45:06 浏览: 48
以下是一个简单的宠物信息管理系统的 C++ 源代码,实现了管理员的所有要求功能,以及用户的姓名和手机号查询宠物信息功能。由于没有具体的界面,所以采用了控制台输出的方式。
```cpp
#include <iostream>
#include <fstream>
#include <vector>
#include <algorithm>
using namespace std;
// 基类: 宠物
class Pet {
public:
virtual ~Pet() {}
virtual void input() = 0; // 录入宠物信息
virtual void output() = 0; // 输出宠物信息
virtual void modify() = 0; // 修改宠物信息
virtual int getID() const = 0; // 获取宠物编号
virtual string getName() const = 0; // 获取宠物名字
};
// 派生类: 狗
class Dog : public Pet {
private:
int id; // 编号
string name; // 名字
string gender; // 性别
int age; // 年龄
string color; // 颜色
string ownerName; // 客户姓名
string ownerPhone; // 客户手机号
public:
Dog() {}
Dog(int id, const string& name, const string& gender, int age, const string& color, const string& ownerName, const string& ownerPhone)
: id(id), name(name), gender(gender), age(age), color(color), ownerName(ownerName), ownerPhone(ownerPhone) {}
void input() override {
cout << "请输入宠物编号: ";
cin >> id;
cout << "请输入宠物名字: ";
cin >> name;
cout << "请输入宠物性别: ";
cin >> gender;
cout << "请输入宠物年龄: ";
cin >> age;
cout << "请输入宠物颜色: ";
cin >> color;
cout << "请输入客户姓名: ";
cin >> ownerName;
cout << "请输入客户手机号: ";
cin >> ownerPhone;
}
void output() override {
cout << "宠物编号: " << id << endl;
cout << "宠物名字: " << name << endl;
cout << "宠物性别: " << gender << endl;
cout << "宠物年龄: " << age << endl;
cout << "宠物颜色: " << color << endl;
cout << "客户姓名: " << ownerName << endl;
cout << "客户手机号: " << ownerPhone << endl;
}
void modify() override {
cout << "请输入新的宠物名字: ";
cin >> name;
cout << "请输入新的宠物性别: ";
cin >> gender;
cout << "请输入新的宠物年龄: ";
cin >> age;
cout << "请输入新的宠物颜色: ";
cin >> color;
cout << "请输入新的客户姓名: ";
cin >> ownerName;
cout << "请输入新的客户手机号: ";
cin >> ownerPhone;
}
int getID() const override {
return id;
}
string getName() const override {
return name;
}
};
// 宠物信息管理系统
class PetManagementSystem {
private:
vector<Dog> dogs; // 所有狗狗的信息
public:
// 管理员菜单
void adminMenu() {
int choice;
while (true) {
cout << "请选择操作: " << endl;
cout << "1. 录入宠物信息" << endl;
cout << "2. 修改宠物信息" << endl;
cout << "3. 浏览宠物信息" << endl;
cout << "4. 删除宠物信息" << endl;
cout << "5. 模糊查询宠物信息" << endl;
cout << "6. 按编号大小排序宠物信息" << endl;
cout << "7. 返回上一级菜单" << endl;
cin >> choice;
switch (choice) {
case 1:
addDog();
break;
case 2:
modifyDog();
break;
case 3:
viewDogs();
break;
case 4:
deleteDog();
break;
case 5:
searchDogs();
break;
case 6:
sortDogs();
break;
case 7:
return;
default:
cout << "无效的选择,请重新输入!" << endl;
}
}
}
// 添加一只狗狗的信息
void addDog() {
Dog dog;
dog.input();
dogs.push_back(dog);
cout << "宠物信息已保存!" << endl;
}
// 修改一只狗狗的信息
void modifyDog() {
int id;
cout << "请输入要修改的宠物编号: ";
cin >> id;
for (auto& dog : dogs) {
if (dog.getID() == id) {
dog.modify();
cout << "宠物信息已修改!" << endl;
return;
}
}
cout << "未找到编号为 " << id << " 的宠物信息!" << endl;
}
// 浏览所有狗狗的信息
void viewDogs() {
if (dogs.empty()) {
cout << "当前没有宠物信息!" << endl;
return;
}
for (auto& dog : dogs) {
dog.output();
cout << endl;
}
}
// 删除一只狗狗的信息
void deleteDog() {
int id;
cout << "请输入要删除的宠物编号: ";
cin >> id;
for (auto iter = dogs.begin(); iter != dogs.end(); iter++) {
if (iter->getID() == id) {
dogs.erase(iter);
cout << "宠物信息已删除!" << endl;
return;
}
}
cout << "未找到编号为 " << id << " 的宠物信息!" << endl;
}
// 模糊查询狗狗的信息
void searchDogs() {
string keyword;
cout << "请输入要查询的关键字: ";
cin >> keyword;
int count = 0;
for (auto& dog : dogs) {
if (dog.getName().find(keyword) != string::npos || dog.ownerName.find(keyword) != string::npos || dog.ownerPhone.find(keyword) != string::npos) {
dog.output();
cout << endl;
count++;
}
}
if (count == 0) {
cout << "未找到与关键字 \"" << keyword << "\" 相关的宠物信息!" << endl;
}
}
// 按编号大小排序狗狗的信息
void sortDogs() {
sort(dogs.begin(), dogs.end(), [](const Dog& a, const Dog& b) {
return a.getID() < b.getID();
});
cout << "宠物信息已按编号大小排序!" << endl;
}
// 用户菜单
void userMenu() {
string name, phone;
cout << "请输入您的姓名: ";
cin >> name;
cout << "请输入您的手机号: ";
cin >> phone;
int count = 0;
for (auto& dog : dogs) {
if (dog.ownerName == name || dog.ownerPhone == phone) {
dog.output();
cout << endl;
count++;
}
}
if (count == 0) {
cout << "未找到与您相关的宠物信息!" << endl;
}
}
// 运行宠物信息管理系统
void run() {
int choice;
while (true) {
cout << "请选择身份: " << endl;
cout << "1. 管理员" << endl;
cout << "2. 用户" << endl;
cout << "3. 退出" << endl;
cin >> choice;
switch (choice) {
case 1:
adminMenu();
break;
case 2:
userMenu();
break;
case 3:
return;
default:
cout << "无效的选择,请重新输入!" << endl;
}
}
}
};
int main() {
PetManagementSystem system;
system.run();
return 0;
}
```
阅读全文
相关推荐





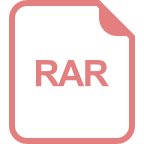
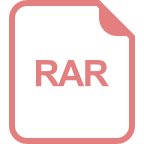




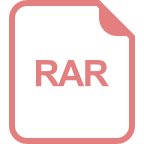





