#include <stdio.h> #include <stdlib.h> typedef char ElementType; typedef struct TNode *Position; typedef Position BinTree; struct TNode{ ElementType Data; BinTree Left; BinTree Right; }; BinTree CreatBinTree(); /* 实现细节忽略 */ int GetHeight( BinTree BT ); void InorderTraversal( BinTree BT ); //中序遍历 void PreorderTraversal( BinTree BT ); //前序遍历 void PostorderTraversal( BinTree BT ); //后序遍历 void LevelorderTraversal( BinTree BT); //水平遍历 int main() { BinTree BT = CreatBinTree(); printf("二叉树的高度:%d\n", GetHeight(BT)); printf("Inorder:"); InorderTraversal(BT); printf("\n"); printf("Preorder:"); PreorderTraversal(BT); printf("\n"); printf("Postorder:"); PostorderTraversal(BT); printf("\n"); printf("Levelorder:"); LevelorderTraversal(BT); printf("\n"); return 0; }补全代码
时间: 2024-01-29 08:02:51 浏览: 80
#include <stdio.h>
#include <stdlib.h>
typedef char ElementType;
typedef struct TNode *Position;
typedef Position BinTree;
struct TNode{
ElementType Data;
BinTree Left;
BinTree Right;
};
BinTree CreatBinTree(); /* 实现细节忽略 */
int GetHeight( BinTree BT );
void InorderTraversal( BinTree BT ); //中序遍历
void PreorderTraversal( BinTree BT ); //前序遍历
void PostorderTraversal( BinTree BT ); //后序遍历
void LevelorderTraversal( BinTree BT); //水平遍历
int main() {
BinTree BT = CreatBinTree();
printf("二叉树的高度:%d\n", GetHeight(BT));
printf("Inorder:");
InorderTraversal(BT);
printf("\n");
printf("Preorder:");
PreorderTraversal(BT);
printf("\n");
printf("Postorder:");
PostorderTraversal(BT);
printf("\n");
printf("Levelorder:");
LevelorderTraversal(BT);
printf("\n");
return 0;
}
int GetHeight( BinTree BT ){
if(!BT){
return 0;
}
int left = GetHeight(BT->Left);
int right = GetHeight(BT->Right);
return left > right ? (left + 1) : (right + 1);
}
void InorderTraversal( BinTree BT ){
if(BT){
InorderTraversal(BT->Left);
printf(" %c", BT->Data);
InorderTraversal(BT->Right);
}
}
void PreorderTraversal( BinTree BT ){
if(BT){
printf(" %c", BT->Data);
PreorderTraversal(BT->Left);
PreorderTraversal(BT->Right);
}
}
void PostorderTraversal( BinTree BT ){
if(BT){
PostorderTraversal(BT->Left);
PostorderTraversal(BT->Right);
printf(" %c", BT->Data);
}
}
void LevelorderTraversal( BinTree BT){
BinTree queue[1000];
int front = -1, rear = -1;
if(BT){
queue[++rear] = BT;
}
while(front < rear){
BinTree temp = queue[++front];
printf(" %c", temp->Data);
if(temp->Left){
queue[++rear] = temp->Left;
}
if(temp->Right){
queue[++rear] = temp->Right;
}
}
}
阅读全文
相关推荐



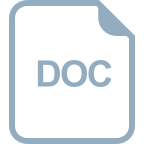














