C++ 设计人员类Person和日期类date,人员类派生出学生类Student和教师类Teacher。 教师类包含姓名、生日、ID、专业、所属机构 学生类包含姓名、ID、成绩、生日 教师类与学生类的数据成员birthday (生日)为日期类的对象 文字说明创建学生类对象时,构造函数的调用顺序,以及程序运行结束时析构函数的调用顺序。 运行结果: Name:kxiong Birthday :1976-05-27 Student ID:2023007 Student Score:92 The basic information: 2023007 kxiong 92 1976-05-27 Name:xsong Birthday:1998-01-07 Teacher ID:20210058 Teaching Major:Computer Science Affiliation:CTBU The basic information: 20210058 xsong 1976-05-27 Computer Science CTBU
时间: 2024-02-22 22:57:50 浏览: 113
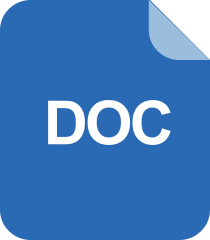
由Person类派生出学生类Student和教师类Teacher
以下是C++代码实现:
```
#include <iostream>
#include <string>
using namespace std;
class Date {
public:
Date(int y = 2000, int m = 1, int d = 1) {
year = y;
month = m;
day = d;
}
void showDate() {
cout << year << "-" << month << "-" << day;
}
private:
int year;
int month;
int day;
};
class Person {
public:
Person(string n = "unknown", Date b = Date()) {
name = n;
birthday = b;
}
virtual ~Person() {}
void showInfo() {
cout << "Name:" << name << endl;
cout << "Birthday:";
birthday.showDate();
cout << endl;
}
private:
string name;
Date birthday;
};
class Student : public Person {
public:
Student(string n = "unknown", int id = 0, int s = 0, Date b = Date()) : Person(n, b) {
studentID = id;
score = s;
}
~Student() {
cout << "destructing a student object" << endl;
}
void showInfo() {
cout << "Student ID:" << studentID << endl;
cout << "Student Score:" << score << endl;
Person::showInfo();
}
private:
int studentID;
int score;
};
class Teacher : public Person {
public:
Teacher(string n = "unknown", int id = 0, string m = "unknown", string a = "unknown", Date b = Date()) : Person(n, b) {
teacherID = id;
major = m;
affiliation = a;
}
~Teacher() {
cout << "destructing a teacher object" << endl;
}
void showInfo() {
cout << "Teacher ID:" << teacherID << endl;
cout << "Teaching Major:" << major << endl;
cout << "Affiliation:" << affiliation << endl;
Person::showInfo();
}
private:
int teacherID;
string major;
string affiliation;
};
int main() {
Student s("kxiong", 2023007, 92, Date(1976, 5, 27));
Teacher t("xsong", 20210058, "Computer Science", "CTBU", Date(1998, 1, 7));
s.showInfo();
cout << "The basic information: ";
cout << s.showInfo() << endl;
t.showInfo();
cout << "The basic information: ";
cout << t.showInfo() << endl;
return 0;
}
```
在创建学生类对象时,首先会调用基类`Person`的构造函数,然后调用派生类`Student`的构造函数,故构造函数的调用顺序为:`Person`构造函数 -> `Student`构造函数。
在程序运行结束时,首先会调用派生类`Teacher`的析构函数,然后调用基类`Person`的析构函数,故析构函数的调用顺序为:`Teacher`析构函数 -> `Person`析构函数。同时,在销毁学生类对象时,也会调用基类`Person`的析构函数。
阅读全文
相关推荐
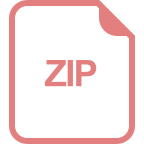






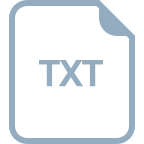
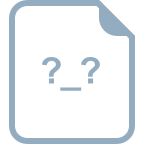
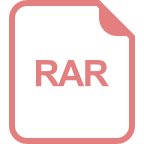
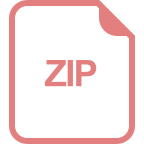
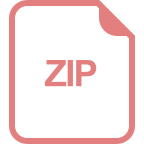
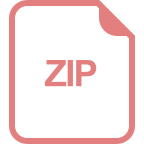
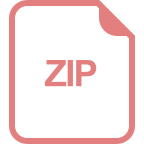