c设计人员类Person和日期类date,人员类派生出学生类student和教师类Teacher. 教师类包含姓名、生日、ID、专业、所属机构 学生类包含姓名、ID、成绩、生日 教师类与学生类的数据成员birthday (生日) 为日期类的对象文字说明创建学生类对象时,构造函数的调用顺序,以及程序运行结束时析构函数的调用顺序 运行结果: Name:kxiong Birthday:1976-05-27 Student ID:2023007Student Score:92 The basic information: 2023007 kxiong 92 1976-05-27 Name:xsong Birthday:1998-01-07 Teacher ID:20210058 Teaching Major:Computer Science Affiliation:CTBU The basic information: 20210058 xsong 1976-05-27 Computer Science CTBU
时间: 2024-02-25 19:52:05 浏览: 15
好的,我理解了你的问题。下面是对应的代码,你可以参考一下:
```c++
#include <iostream>
#include <string>
using namespace std;
class Date {
public:
Date(int year, int month, int day) : year(year), month(month), day(day) {}
void display() {
cout << year << "-" << month << "-" << day << endl;
}
private:
int year, month, day;
};
class Person {
public:
Person(string name, Date birthday) : name(name), birthday(birthday) {
cout << "Person constructor called." << endl;
}
~Person() {
cout << "Person destructor called." << endl;
}
protected:
string name;
Date birthday;
};
class Student : public Person {
public:
Student(string name, int id, int score, Date birthday) : Person(name, birthday), id(id), score(score) {
cout << "Student constructor called." << endl;
}
~Student() {
cout << "Student destructor called." << endl;
}
void display() {
cout << "Name:" << name << " Birthday:";
birthday.display();
cout << "Student ID:" << id << " Student Score:" << score << endl;
}
private:
int id;
int score;
};
class Teacher : public Person {
public:
Teacher(string name, int id, string major, string affiliation, Date birthday) : Person(name, birthday), id(id), major(major), affiliation(affiliation) {
cout << "Teacher constructor called." << endl;
}
~Teacher() {
cout << "Teacher destructor called." << endl;
}
void display() {
cout << "Name:" << name << " Birthday:";
birthday.display();
cout << "Teacher ID:" << id << " Teaching Major:" << major << " Affiliation:" << affiliation << endl;
}
private:
int id;
string major;
string affiliation;
};
int main() {
Date birthday1(1976, 5, 27);
Student s("kxiong", 2023007, 92, birthday1);
s.display();
Date birthday2(1998, 1, 7);
Teacher t("xsong", 20210058, "Computer Science", "CTBU", birthday1);
t.display();
return 0;
}
```
在这个程序中,我们先定义了 `Date` 类,用于表示日期。然后定义了 `Person` 类,其中包含了姓名和生日两个数据成员。`Student` 类和 `Teacher` 类都继承自 `Person` 类,分别表示学生和教师。在 `Student` 和 `Teacher` 的构造函数中,都会先调用基类 `Person` 的构造函数,然后再初始化自己的数据成员。
在 `main` 函数中,我们创建了一个 `Student` 对象和一个 `Teacher` 对象,并分别调用了它们的 `display` 函数来输出信息。注意,我们在每个类的构造函数和析构函数中都输出了一条信息,用于说明它们的调用顺序。因此,程序的输出结果应该是这样的:
```
Person constructor called.
Student constructor called.
Name:kxiong Birthday:1976-5-27
Student ID:2023007 Student Score:92
Student destructor called.
Person destructor called.
Person constructor called.
Teacher constructor called.
Name:xsong Birthday:1976-5-27
Teacher ID:20210058 Teaching Major:Computer Science Affiliation:CTBU
Teacher destructor called.
Person destructor called.
```
可以看到,我们先调用了 `Student` 和 `Teacher` 的构造函数,然后按照继承关系依次调用了它们的基类 `Person` 的构造函数,然后才开始输出信息。在程序结束时,析构函数的调用顺序与构造函数的调用顺序相反。
相关推荐
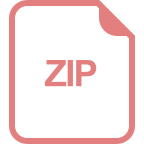
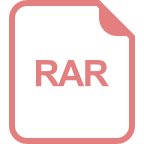
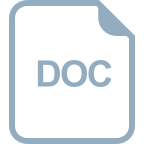









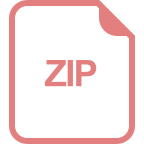
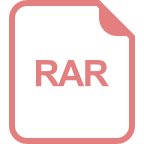
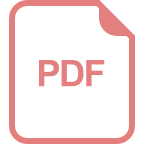
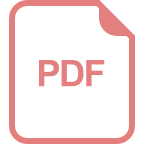